Attach event handlers inside other handlers
Written byPhuoc Nguyen
Created
24 Apr, 2020
Category
Tip
Usually, there are many event handlers which handle different events for different elements. These events could depend on each other.
Let's look at a common use case. When user clicks a button, we will open a modal at the center of screen. The modal can be closed by pressing the Escape key.
There are two handlers here:
- The first one handles the
`click`
event of the button - The second one handles the
`keydown`
event of the entire`document`
We often create two separated handlers as following:
js
const handleClick = function () {
// Open the modal
};
const handleKeydown = function (e) {
// Close the modal if the Escape key is pressed
};
// Assume that `buttonEle` represents the button element
buttonEle.addEventListener('click', handleClick);
document.addEventListener('keydown', handleKeydown);
The
`handleKeydown`
handler depends on `handleClick`
because we only check the pressed key if the modal is already opened.
It's a common way to add a flag to track if the modal is opened or not:js
let isModalOpened = false;
const handleClick = function () {
// Turn on the flag
isModalOpened = true;
// Open the modal ...
};
const handleKeydown = function (e) {
// Check if the modal is opened first
if (isModalOpened) {
// Check the pressed key ...
}
};
More elements, more dependent events and more flags! As the result, it's more difficult to maintain the code.
Instead of adding event separately at first, we add an event handler right inside another one which it depends on.
Here is how the tip approaches:
js
const handleClick = function () {
document.addEventListener('keydown', handleKeydown);
};
No flag at all! The code is more readable and easier to understand.
#Use cases
You can see the tip used in another posts:
- Create a custom scrollbar
- Create a range slider
- Create an image comparison slider
- Create resizable split views
- Drag to scroll
- Make a draggable element
- Make a resizable element
- Print an image
- Resize columns of a table
- Show a custom context menu at clicked position
- Show or hide table columns
#See also
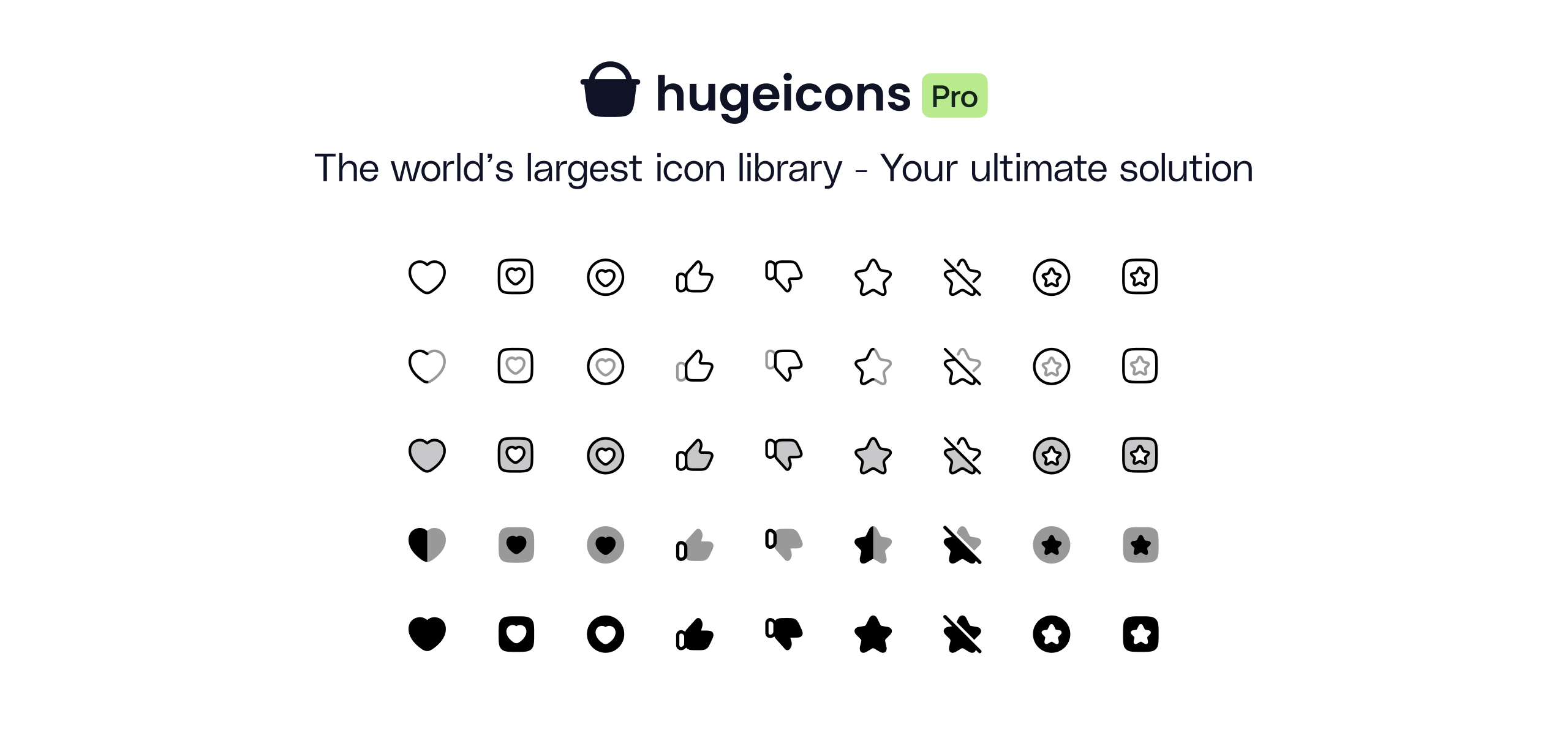
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn