Clipboard API fallback
Written byPhuoc Nguyen
Created
20 Jan, 2024
Tags
Clipboard API, document.execCommand
In our previous post, we learned how to use feature detection to check if the Clipboard API is supported. However, in cases where it isn't supported, we can use a fallback. One of the alternatives is the
`document.execCommand`
method.To use
`document.execCommand`
as a fallback when the Clipboard API is not supported, here's an example:js
if (navigator.clipboard) {
// Clipboard API is supported
} else {
// Clipboard API is not supported
document.execCommand('copy');
}
#Understanding document.execCommand method
The
`document.execCommand`
method is a method that developers can use to manipulate clipboard content. This method has been around since the early days of web development and is built into all modern browsers.The
`document.execCommand`
method takes two arguments: a command identifier and a Boolean value that determines whether to show UI for the command. Here's an example:js
document.execCommand('copy', false);
Here are some common commands you might encounter while using text editors:
`copy`
: copies the selected text to the clipboard.`cut`
: cuts the selected text and stores it in the clipboard.`paste`
: pastes the content from clipboard to the current cursor position.`bold`
: makes selected text bold.`italic`
: makes selected text italic.
Want to see how these commands work in action? Check out this example that demonstrates using the
`execCommand`
method to copy text to the clipboard.js
const writeToClipboard = (text) => {
const textArea = document.createElement("textarea");
textArea.value = text;
// Styling to hide the textarea
textArea.style.position = "fixed";
textArea.style.top = "-9999px";
textArea.style.left = "-9999px";
document.body.appendChild(textArea);
textArea.focus();
textArea.select();
try {
const successful = document.execCommand("copy");
const message = successful ? "successful" : "unsuccessful";
console.log(`Copying text was ${message}`);
} catch (error) {
console.error("Unable to copy to clipboard", error);
}
document.body.removeChild(textArea);
};
The
`writeToClipboard`
function in the code above is a custom function that copies text to your clipboard.First, the function creates a new text area element using the
`document.createElement`
method. The text to be copied is set as the value of this element.Next, the function applies styling to hide the text area from view by setting its position to
`fixed`
and its top and left values to `-9999px`
. This ensures that the text area is not visible on the screen but can still be accessed.The newly created text area element is then added to the document body using
`document.body.appendChild(textArea)`
.The function then calls the
`focus`
method on the text area and selects all of its content using the `select`
method.Finally, the
`document.execCommand('copy')`
method is called, which copies the selected text to your clipboard. Any errors are caught by a try-catch block, and if there are no errors, the function removes the temporary text area from the document using `document.body.removeChild(textArea)`
.#Comparing document.execCommand and Clipboard API
When it comes to copying and pasting data, it's important to choose the right tool for the job. While both
`document.execCommand`
and the Clipboard API can be used for this purpose, they have some key differences that are worth noting.One of the most significant differences is that
`document.execCommand`
is a synchronous method, meaning it can cause performance issues and slower page load times. On the other hand, the Clipboard API is asynchronous, which makes it a better choice for handling large or complex data sets.Security is another important consideration.
`document.execCommand`
requires elevated permissions to execute certain commands, which can make it more difficult to use in some contexts - especially on mobile devices where users are less likely to grant permission for elevated access. The Clipboard API, on the other hand, provides a more secure way to access and modify clipboard data by requiring explicit user permission.While
`document.execCommand`
may still be useful as a fallback option in some cases, developers should prioritize using the Clipboard API whenever possible due to its improved performance and security features.#Copying vs cutting text
Copying and cutting text are two common tasks that developers need to perform when building web applications. While both
`document.execCommand('copy')`
and the Clipboard API can be used to copy text, only `document.execCommand`
can be used to cut text.Cutting text is similar to copying text, but instead of copying the selected text to the clipboard, it removes it from the document and saves it to the clipboard. This can be useful when a user wants to move some selected text to a different location within a document or application.
However, it's important to note that just like copying text using
`document.execCommand`
, there are security concerns with cutting text as well. In some browsers, the method can only be executed as a result of a user action such as a click event.On the other hand, the Clipboard API does not provide an explicit method for cutting text. Instead, developers need to use other methods such as manipulating the DOM or using JavaScript's string manipulation functions to remove and store selected text before adding it back in at a later time.
Ultimately, developers should consider their specific use case and browser compatibility requirements when deciding which method to use. While both methods have their pros and cons when it comes to copying and cutting text, it's important to choose the one that fits the situation best.
#Security concerns with document.execCommand
One of the major drawbacks of using
`document.execCommand`
is its security concerns. As we mentioned earlier, executing commands such as "cut" or "paste" requires elevated permissions, which can pose a risk to users' security.For example, if an attacker injects malicious code into a webpage that uses
`document.execCommand`
, it can access and modify clipboard data without the user's consent. This can lead to sensitive information being compromised, such as login credentials, credit card numbers, or personal information.Moreover, some web browsers may block the execution of certain commands for security reasons. For instance, the "paste" command may be disabled on mobile devices due to concerns about sensitive data being pasted unintentionally.
To mitigate these risks, developers must use
`document.execCommand`
responsibly and only execute commands when necessary. They should also avoid using this method for sensitive operations such as copying or cutting passwords or other confidential information.Overall, while
`document.execCommand`
can be a useful fallback option in some cases where the Clipboard API is not supported, developers should be aware of its potential security vulnerabilities and use it with caution.#Conclusion
In conclusion, while the
`document.execCommand`
method can be used as a fallback for the Clipboard API, there are several limitations that make it less desirable compared to the Clipboard API.Firstly, the
`document.execCommand`
method is no longer recommended to use because it is deprecated. Secondly, the Clipboard API provides a more secure and reliable way to interact with clipboard content. It is also asynchronous, which prevents blocking of the main thread, and requires explicit user permission before accessing or modifying clipboard data.Developers should prioritize using the Clipboard API whenever possible due to its improved performance and security features. However, there may be cases where
`document.execCommand`
is still useful, such as when the Clipboard API is not supported or when cutting text is required.It's essential for developers to use these methods responsibly and only execute commands when necessary, while avoiding using them for sensitive operations. By doing so, we can ensure that our applications are secure and provide a better user experience.
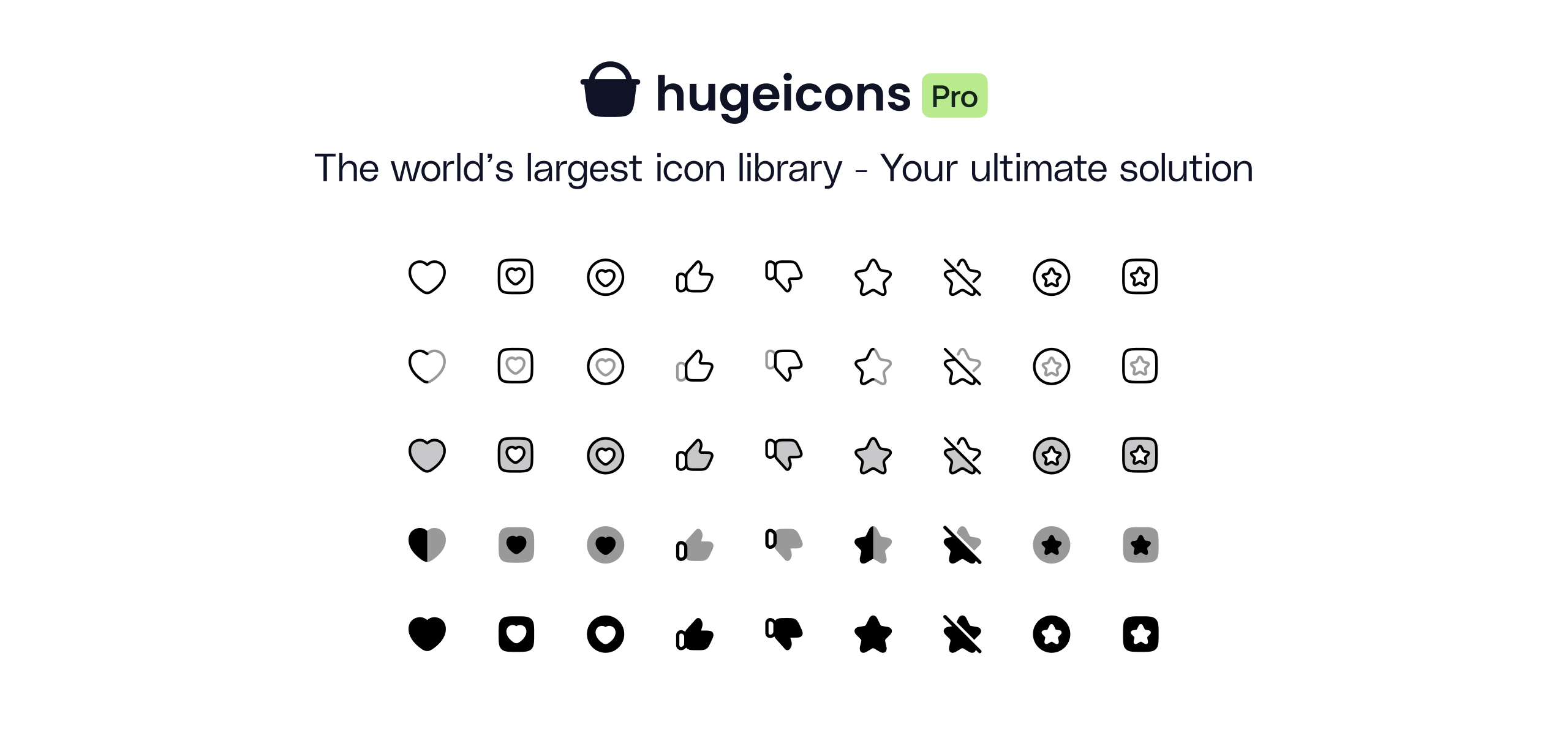
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn