Check if the Clipboard API is supported
Written byPhuoc Nguyen
Created
19 Jan, 2024
Tags
Clipboard API, feature detection
If you're creating a web application that relies on the Clipboard API, it's crucial to determine whether the user's browser supports the API. Here's a quick and easy way to check if the Clipboard API is available:
#Using the navigator.clipboard property
To check if the Clipboard API is available, you can simply check the
`navigator.clipboard`
property. If the Clipboard API is supported, this property will return a `Clipboard`
object. Otherwise, it will return `undefined`
.js
if (navigator.clipboard) {
// Clipboard API is supported
} else {
// Clipboard API is not supported
}
#Using feature detection
Even if a browser supports the Clipboard API, not all of its methods are supported. In such cases, you can use feature detection to check the availability of the Clipboard API.
Feature detection is a technique that helps you determine whether a web browser or device supports a specific feature, API, or technology. Instead of relying on browser user-agent strings or assumptions about the environment, you can use feature detection to test for the availability of specific features and adjust your code accordingly.
To use feature detection, you typically check for the existence of specific properties or methods on objects such as
`navigator`
or `window`
. By checking if these properties and methods exist before using them in your code, you can avoid errors and ensure that your application works as expected on all devices and browsers.In the context of Clipboard API support, you might use feature detection to determine which methods are available for reading and writing clipboard data. This way, you can provide fallbacks or alternative behavior for browsers that don't fully support the API.
Here's an example:
js
const isClipboardApiSupported = () => {
return !!(
navigator.clipboard &&
navigator.clipboard.readText &&
navigator.clipboard.writeText
);
};
if (isClipboardApiSupported()) {
// Clipboard API is supported
} else {
// Clipboard API is not supported
}
This function checks if the
`navigator.clipboard`
property exists and if its `readText`
and `writeText`
methods are available. If all these conditions are true, then the Clipboard API is supported. You might want to use more checks to suit with your specific requirement.By checking if the Clipboard API is available before using it in your web application, you can ensure that your application works as expected on all browsers.
#Browser support for the Clipboard API
It's worth noting that not all browsers support the Clipboard API consistently. While most modern browsers do, some older browsers do not.
As of writing this, caniuse.com reports good support for this feature among popular desktop and mobile browsers including Chrome, Firefox, Safari, and Edge. Unfortunately, Internet Explorer does not support the Clipboard API, but let's be honest, who cares about that browser anymore?
As a web developer, it's important to keep in mind which browsers support the Clipboard API when building your application. You may need to include fallbacks or alternative methods of functionality for browsers that do not support this feature.
In the next post, we'll explore one of these fallback methods.
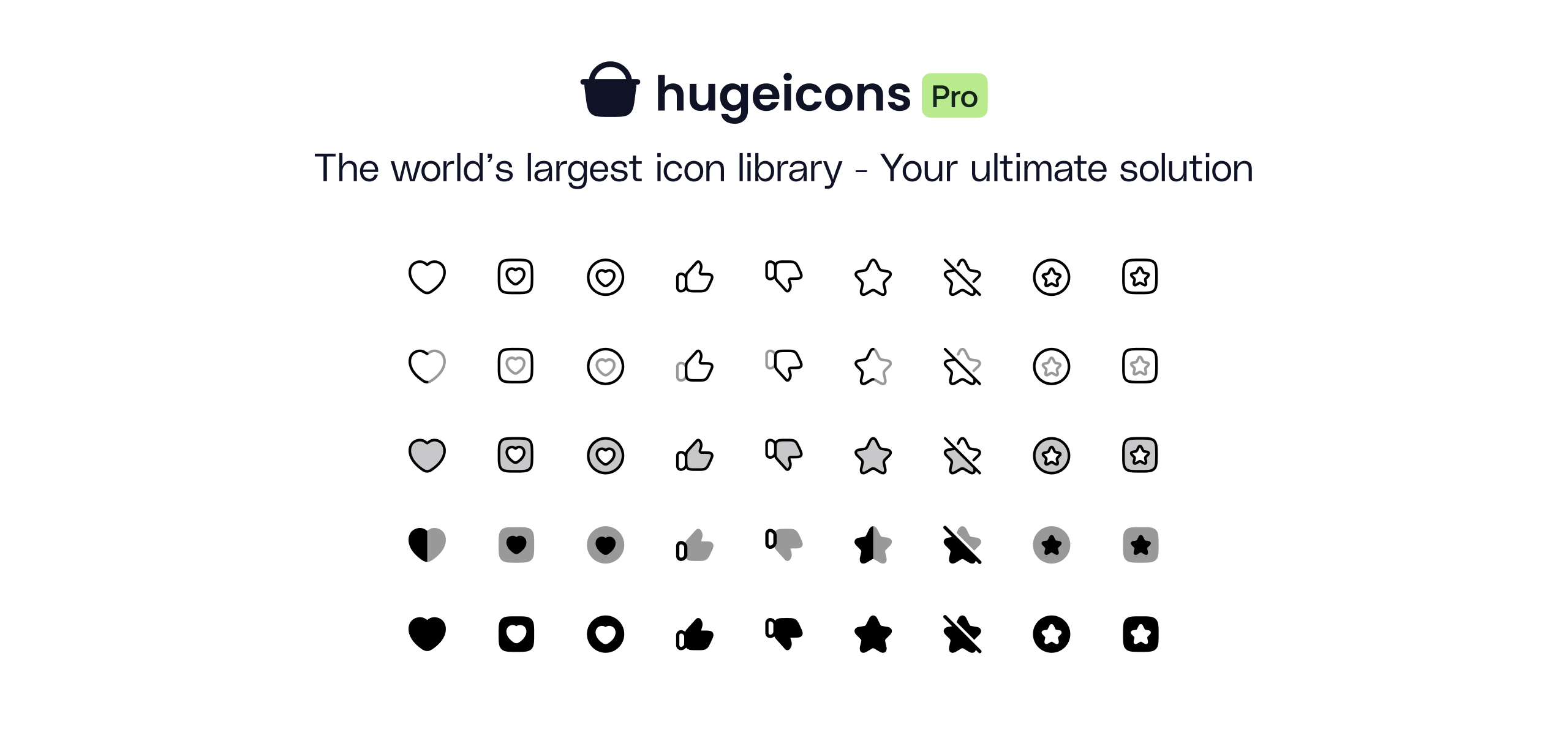
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn