Drag to scroll
Written byPhuoc Nguyen
Created
20 Apr, 2020
Last updated
16 Sep, 2021
Category
Level 3 — Advanced
User often uses the mouse to scroll in a scrollable container. In addition to that, some applications also allow user to scroll by dragging the element. You can see that feature implemented in a PDF viewer, Figma and many more.
This post shows you a simple way to archive that feature with vanilla JavaScript.
Assume that we have a scrollable container as below:
html
<div id="container" class="container">...</div>
The element must have at least two CSS properties:
css
.container {
cursor: grab;
overflow: auto;
}
The
`cursor: grab`
indicates that the element can be clicked and dragged.#Scroll to given position
As long as the element is scrollable, we can scroll it to given position by setting the
`scrollTop`
or `scrollLeft`
property:js
const ele = document.getElementById('container');
ele.scrollTop = 100;
ele.scrollLeft = 150;
#Drag to scroll
The implementation is quite straightforward. By using the similar technique in the Make a draggable element post, we start with handling the
`mousedown`
event which occurs when user clicks the element:js
let pos = { top: 0, left: 0, x: 0, y: 0 };
const mouseDownHandler = function (e) {
pos = {
// The current scroll
left: ele.scrollLeft,
top: ele.scrollTop,
// Get the current mouse position
x: e.clientX,
y: e.clientY,
};
document.addEventListener('mousemove', mouseMoveHandler);
document.addEventListener('mouseup', mouseUpHandler);
};
TipThis post uses the Attach event handlers inside other handlers tip
`pos`
stores the current scroll and mouse positions. When user moves the mouse, we calculate how far it has been moved, and then scroll to the element to the same position:js
const mouseMoveHandler = function (e) {
// How far the mouse has been moved
const dx = e.clientX - pos.x;
const dy = e.clientY - pos.y;
// Scroll the element
ele.scrollTop = pos.top - dy;
ele.scrollLeft = pos.left - dx;
};
Good practiceAs you see above, the`left`
,`top`
,`x`
, and`y`
properties are related to each other. It's better to encapsulate them in a single variable`pos`
instead of creating four variables.
Last but not least, we can improve the user experience by setting some CSS properties when user starts moving the mouse:
js
const mouseDownHandler = function(e) {
// Change the cursor and prevent user from selecting the text
ele.style.cursor = 'grabbing';
ele.style.userSelect = 'none';
...
};
These CSS properties are reset when the mouse is released:
js
const mouseUpHandler = function () {
document.removeEventListener('mousemove', mouseMoveHandler);
document.removeEventListener('mouseup', mouseUpHandler);
ele.style.cursor = 'grab';
ele.style.removeProperty('user-select');
};
#Use cases
Hopefully you love the following demo!
#Demo
#See also
- Create a range slider
- Create an image comparison slider
- Create resizable split views
- Drag and drop element in a list
- Drag and drop table column
- Drag and drop table row
- Make a draggable element
- Make a resizable element
- Resize columns of a table
- Show a ghost element when dragging an element
- Zoom an image
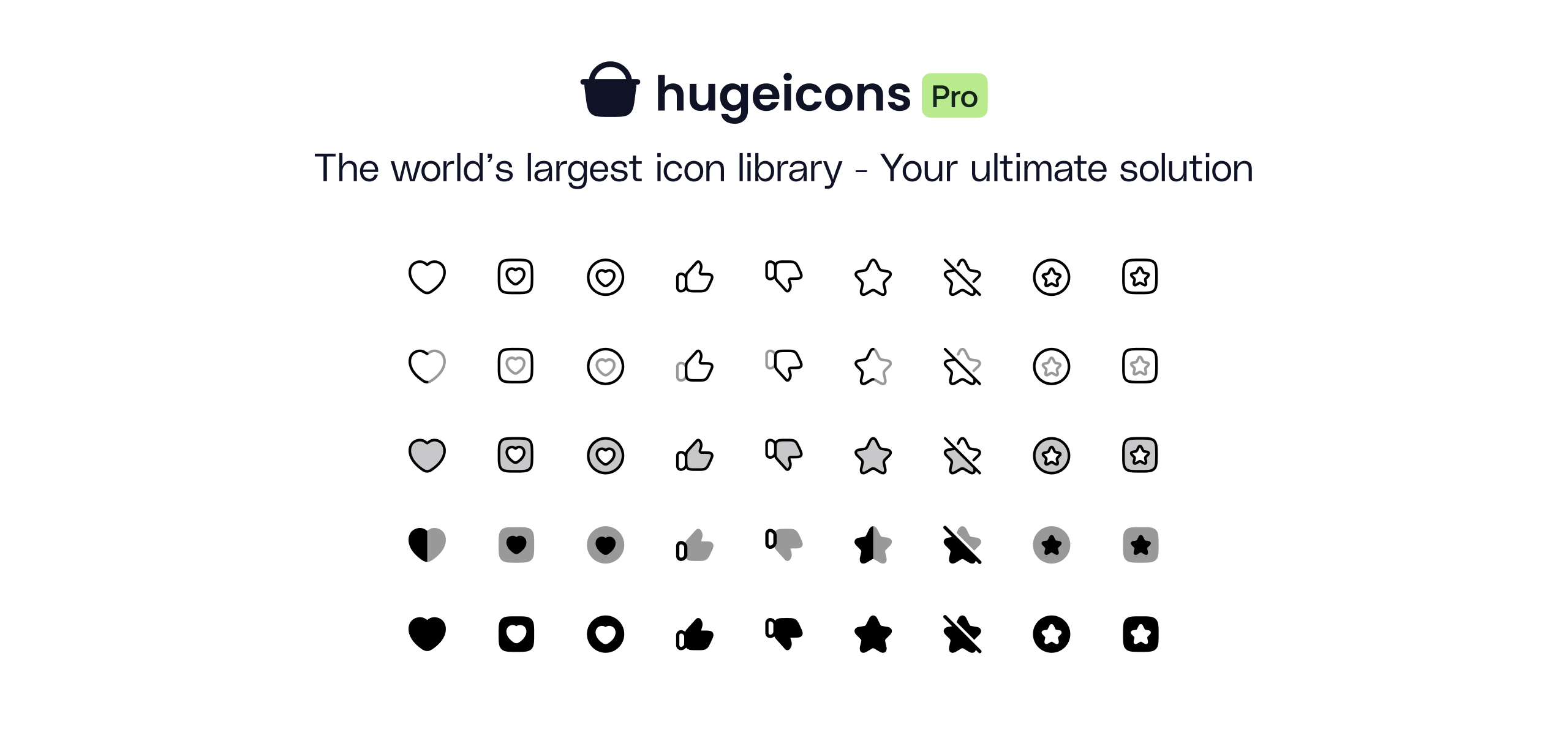
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn