Make a resizable element
Written byPhuoc Nguyen
Created
03 Mar, 2020
Last updated
07 May, 2021
Category
Level 3 — Advanced
Assume that we want to turn the following element to draggable element:
html
<div id="resizeMe" class="resizable">Resize me</div>
First, we need to prepare some elements that indicate the element is resizable. They are placed absolutely at the four sides of the original element.
To make the demo simply, I only prepare two of them which are placed at the right and bottom sides:
html
<div id="resizeMe" class="resizable">
Resize me
<div class="resizer resizer-r"></div>
<div class="resizer resizer-b"></div>
</div>
Here is the basic styles for the layout:
css
.resizable {
position: relative;
}
.resizer {
/* All resizers are positioned absolutely inside the element */
position: absolute;
}
/* Placed at the right side */
.resizer-r {
cursor: col-resize;
height: 100%;
right: 0;
top: 0;
width: 5px;
}
/* Placed at the bottom side */
.resizer-b {
bottom: 0;
cursor: row-resize;
height: 5px;
left: 0;
width: 100%;
}
To make the element resizable, we are going to handle three events:
`mousedown`
on the resizers: Track the current position of mouse and dimension of the original element`mousemove`
on`document`
: Calculate how far the mouse has been moved, and adjust the dimension of the element`mouseup`
on`document`
: Remove the event handlers of`document`
js
// Query the element
const ele = document.getElementById('resizeMe');
// The current position of mouse
let x = 0;
let y = 0;
// The dimension of the element
let w = 0;
let h = 0;
// Handle the mousedown event
// that's triggered when user drags the resizer
const mouseDownHandler = function (e) {
// Get the current mouse position
x = e.clientX;
y = e.clientY;
// Calculate the dimension of element
const styles = window.getComputedStyle(ele);
w = parseInt(styles.width, 10);
h = parseInt(styles.height, 10);
// Attach the listeners to `document`
document.addEventListener('mousemove', mouseMoveHandler);
document.addEventListener('mouseup', mouseUpHandler);
};
const mouseMoveHandler = function (e) {
// How far the mouse has been moved
const dx = e.clientX - x;
const dy = e.clientY - y;
// Adjust the dimension of element
ele.style.width = `${w + dx}px`;
ele.style.height = `${h + dy}px`;
};
const mouseUpHandler = function () {
// Remove the handlers of `mousemove` and `mouseup`
document.removeEventListener('mousemove', mouseMoveHandler);
document.removeEventListener('mouseup', mouseUpHandler);
};
TipThis post uses the Attach event handlers inside other handlers tip
All the event handlers are ready. Finally, we attach the
`mousedown`
event handler to all the resizers:js
// Query all resizers
const resizers = ele.querySelectorAll('.resizer');
// Loop over them
[].forEach.call(resizers, function (resizer) {
resizer.addEventListener('mousedown', mouseDownHandler);
});
#Demo
#See also
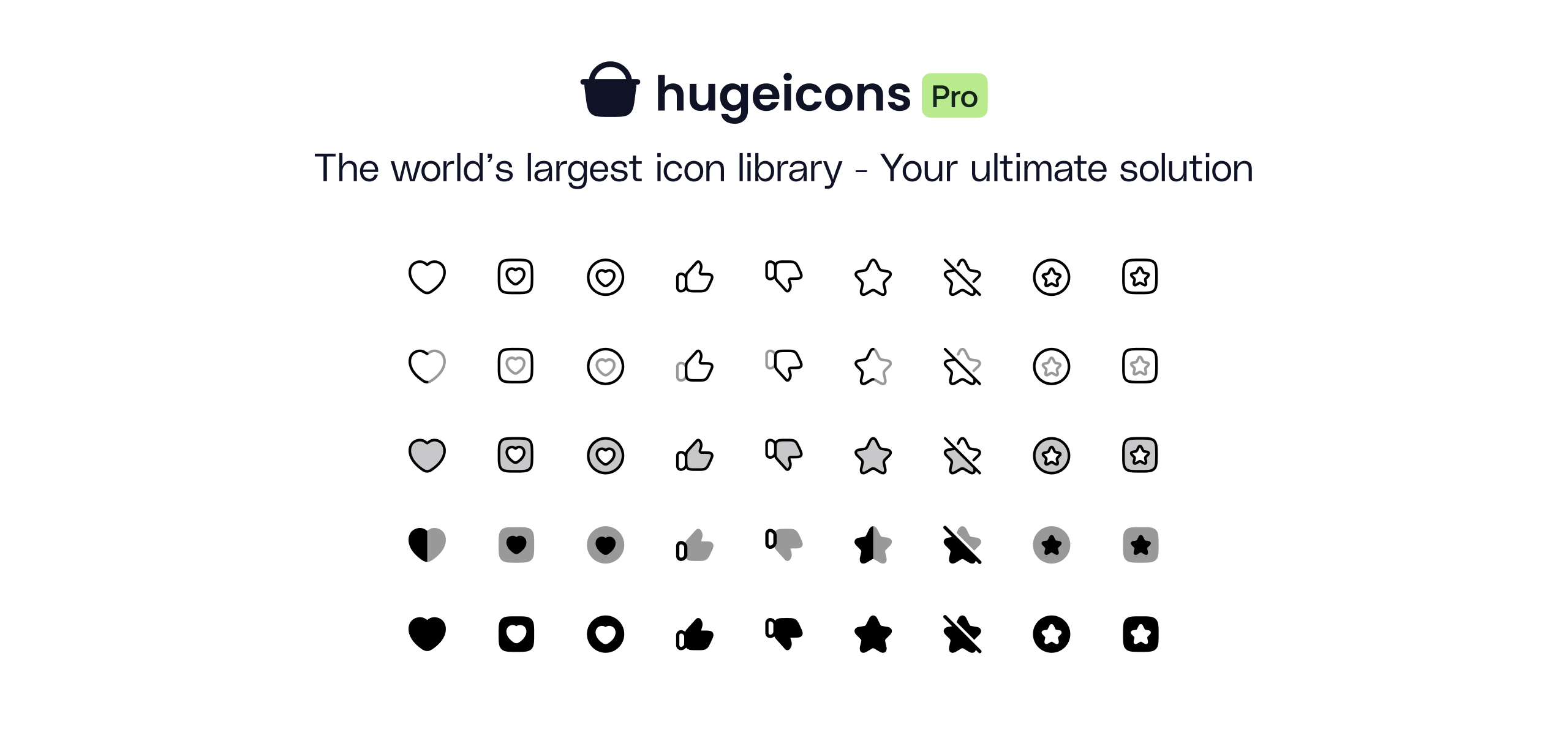
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn