Show a custom context menu at clicked position
Written byPhuoc Nguyen
Created
21 Apr, 2020
Last updated
07 May, 2021
Category
Level 2 — Intermediate
The browser will show the default context menu when user right-clicks on the page. Sometimes, we want to replace the default menu with our own menu that allows user to perform additional actions.
This post illustrates a simple implementation. First of all, let's create an element that we want to show a customized context menu element:
html
<div id="element">Right-click me</div>
<ul id="menu">...</menu>
#Prevent the default context menu from being displayed
To do that, we just prevent the default action of the
`contextmenu`
event:js
const ele = document.getElementById('element');
ele.addEventListener('contextmenu', function (e) {
e.preventDefault();
});
#Show the menu at clicked position
We will calculate the position of menu, but it need to be positioned absolutely to its container firstly. So, let's place the element and menu to a container whose position is
`relative`
:html
<div class="relative">
<div id="element">Right-click me</div>
<ul id="menu" class="absolute hidden">...</menu>
</div>
The
`relative`
, `absolute`
and `hidden`
classes are defined as following:css
.relative {
position: relative;
}
.absolute {
position: absolute;
}
.hidden {
/* The menu is hidden at first */
display: none;
}
ResourceThis post doesn't tell how to build the menu. It's up to you but CSS Layout is a nice resource to look at
It's the time to set the position for the menu. It can be calculated based on the mouse position:
js
ele.addEventListener('contextmenu', function (e) {
const rect = ele.getBoundingClientRect();
const x = e.clientX - rect.left;
const y = e.clientY - rect.top;
// Set the position for menu
menu.style.top = `${y}px`;
menu.style.left = `${x}px`;
// Show the menu
menu.classList.remove('hidden');
});
#Close the menu when clicking outside
js
ele.addEventListener('contextmenu', function(e) {
...
document.addEventListener('click', documentClickHandler);
});
// Hide the menu when clicking outside of it
const documentClickHandler = function(e) {
const isClickedOutside = !menu.contains(e.target);
if (isClickedOutside) {
// Hide the menu
menu.classList.add('hidden');
// Remove the event handler
document.removeEventListener('click', documentClickHandler);
}
};
The menu is hidden by adding the
`hidden`
class.More importantly, the
`click`
event handler is also removed from `document`
as we don't need to handle that when the menu is hidden. This technique is mentioned in the Create one time event handler post.TipThis post uses the Attach event handlers inside other handlers tip
#Use case
Finally, following is the demo you can play with!
#Demo
#See also
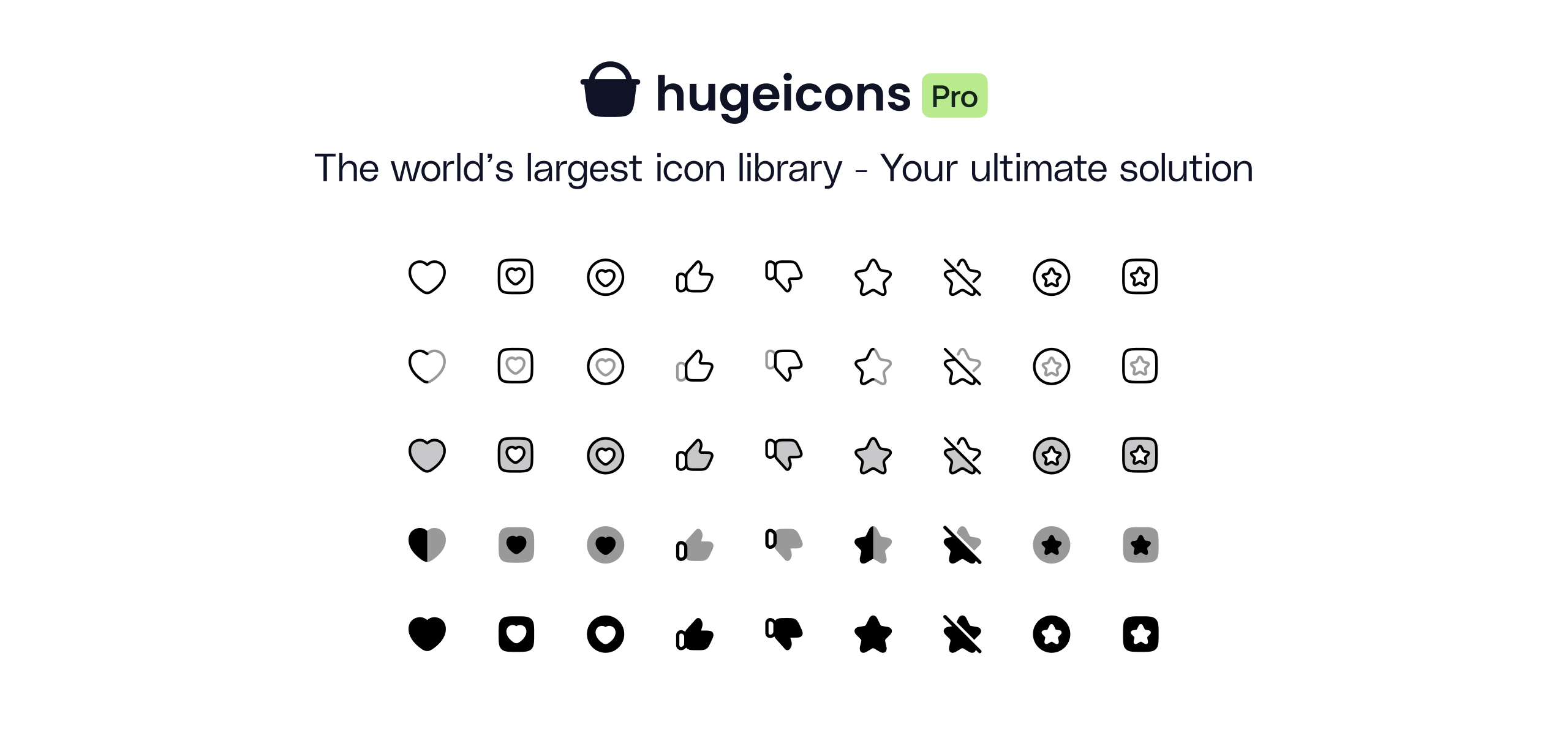
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn