Insert text into a text area at the current position
Written byPhuoc Nguyen
Created
19 Sep, 2023
Category
Level 1 — Basic
If you want to add text to a text area using JavaScript, you can work with the DOM. Check out this handy function called
`insertText()`
below. It only requires two parameters: `textarea`
, which represents the text area element, and `text`
, which is the text you want to insert.js
const insertText = (textarea, text) => {
// Get the current cursor position
const position = textarea.selectionStart;
// Get the text before and after the cursor position
const before = textarea.value.substring(0, position);
const after = textarea.value.substring(position, textarea.value.length);
// Insert the new text at the cursor position
textarea.value = before + text + after;
// Set the cursor position to after the newly inserted text
textarea.selectionStart = textarea.selectionEnd = position + text.length;
};
First, we find the cursor's position by using the
`selectionStart`
property of the text area element. Then, we use the `substring`
method to grab the text before and after the cursor position. With this information, we can insert the new text into the text area at the cursor position. We do this by combining the `before`
, `new text`
, and `after`
variables. Finally, we move the cursor to after the newly inserted text using the `selectionStart`
and `selectionEnd`
properties of the text area element.#Using setRangeText() function
The
`setRangeText()`
method is another way to insert text into a text area. The syntax for this method is as follows:js
textarea.setRangeText(replacement, start, end, select);
The
`replacement`
parameter specifies the new text that will be inserted into the text area. The `start`
parameter specifies the index at which the new text will start being inserted. The `end`
parameter specifies the index at which the new text insertion will end. Finally, the optional `select`
parameter determines whether or not to select the newly inserted text.Using this method is simpler than using
`substring`
, and it can also handle more advanced scenarios such as replacing selected text with new text.Here's another version of the
`insertText()`
function:js
const insertText = (textarea, text) => {
const position = textarea.selectionStart;
const end = position + text.length;
textarea.setRangeText(text, start, end, 'select');
};
#See also
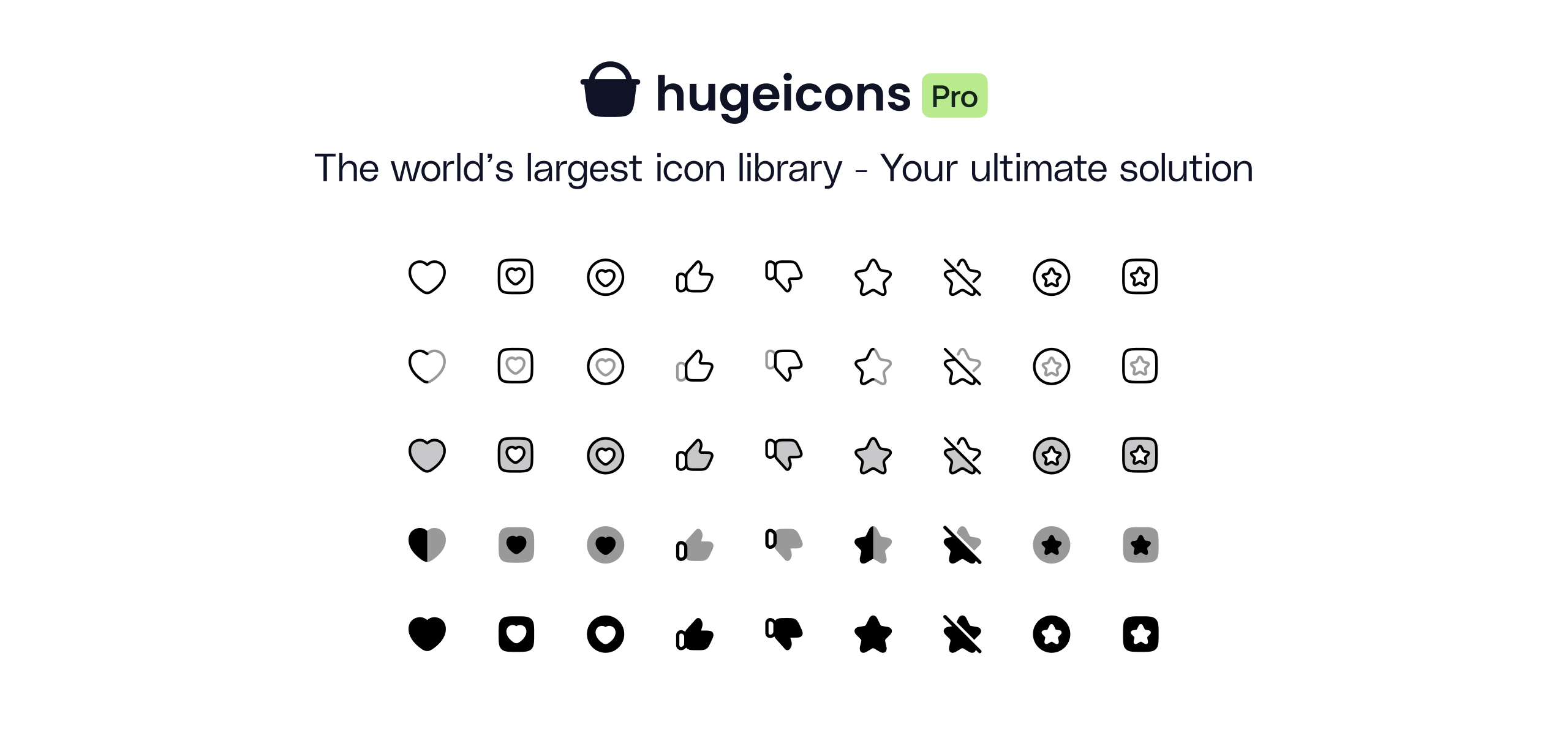
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn