Select a given line in a text area
Written byPhuoc Nguyen
Created
24 Sep, 2023
Category
Level 1 — Basic
Have you ever wanted to select a specific line in a text area using JavaScript DOM? This can come in handy when you need to highlight a particular line of text or copy it to the clipboard.
One real-life example where this technique might be useful is in building a code editor. Code editors often have features that allow users to navigate through their code and highlight specific sections of it. By selecting a particular line, we can help users better visualize and understand their code, leading to a more seamless coding experience. This functionality could also be helpful in creating chat applications where users may want to copy specific lines of conversation for reference or sharing purposes.
In this post, we'll dive into how to implement this functionality with JavaScript.
#Retrieving the text area element
To get started, use the
`document.getElementById()`
method to retrieve the text area element. Simply pass in the ID of the text area element as an argument.js
const textarea = document.getElementById('textarea');
#Retrieving the beginning and ending indices of the line
If you want to choose a particular line of text in the text area, you'll need to locate the beginning and end indices of that line. To achieve that, we need to retrieve the value of the text area and split it using the newline character (
`\n`
).To find the starting position of the selected line, we first create an array of zeros with the same length as the line's index. Then, we map this array to an array of line lengths (adding one for the newline character). Finally, we add up all the elements before the index of your selected line to get the start position.
To determine the end position of your selection, add the length of the line you selected to its starting point. This will give you the index of the last character in your selection.
In the sample code provided, we're selecting the third line (index 2) of the text area element.
js
const lines = textarea.value.split('\n');
// Select the third line (index 2)
const lineIndex = 2;
const start = Array(lineIndex)
.fill(0)
.map((_, i) => (lines[i].length + 1))
.reduce((a, b) => a + b, 0);
// Add the length of the previous lines and the newline character
const end = start + lines[lineIndex].length;
To simplify the calculation of the starting position, you can use the
`reduce()`
method instead of mapping and reducing. With `reduce()`
, we can add up the lengths of each line up to the selected line's index. Then, we add one more character to account for the newline character.js
const start = lines.slice(0, lineIndex).reduce((acc, curr) => acc + curr.length + 1, 0);
#Selecting the range
To set the selection range of a text area element to the start and end indices of a line, use the
`setSelectionRange()`
method. Just remember to call the `focus()`
function first to ensure the text area element is properly focused before selecting the range.js
textarea.focus();
textarea.setSelectionRange(start, end);
Now, the third line of the text area element should be selected.
#See also
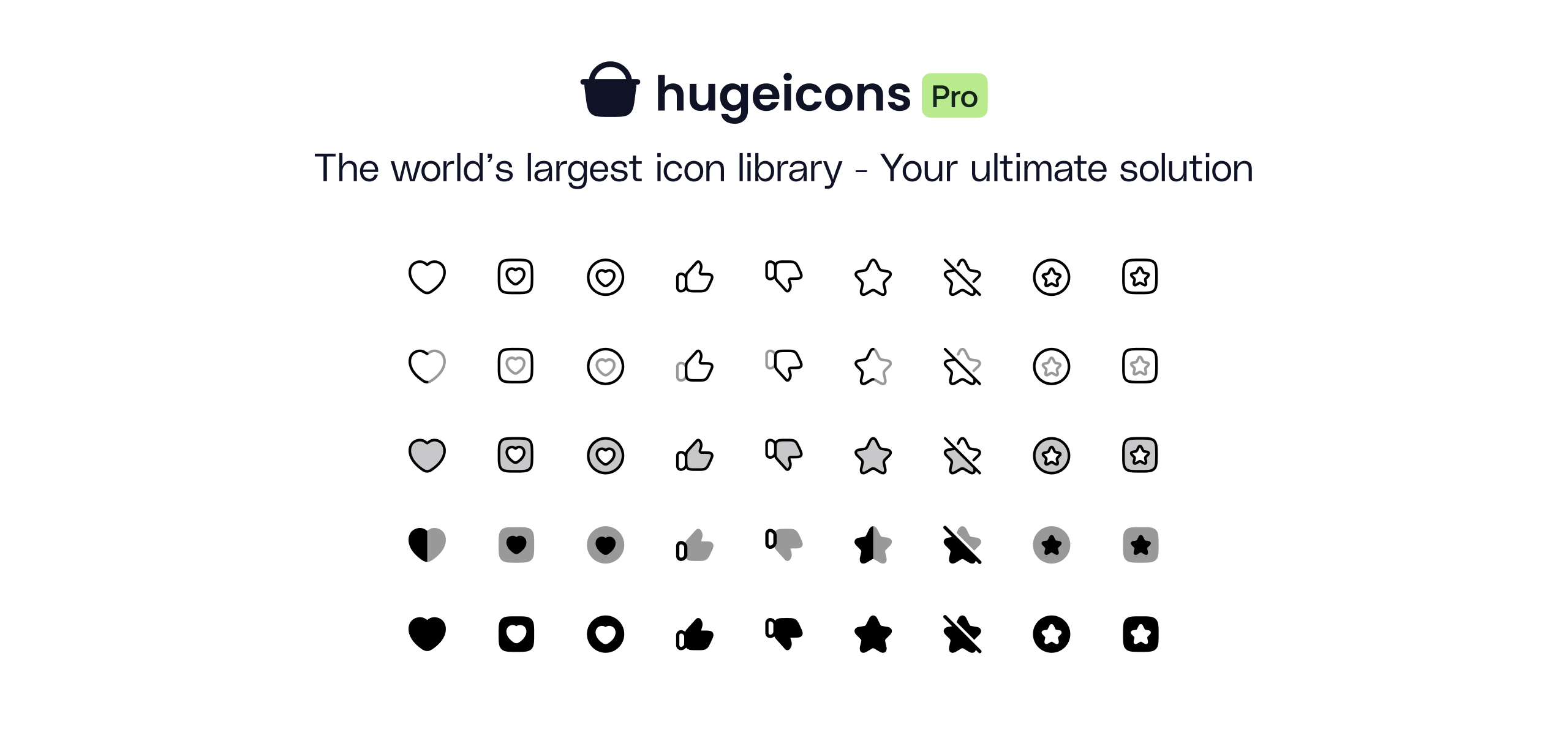
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn