Insert HTML at the current position of a contentEditable element
Written byPhuoc Nguyen
Created
20 Sep, 2023
Category
Level 1 — Basic
When you're working with contentEditable elements in HTML, sometimes you need to insert HTML right where the user is typing using JavaScript.
One real-life example of this is in a WYSIWYG editor. Let's say a user selects some text and clicks on the bold button. The editor can then insert
`<strong>`
tags around the selected text at the current position. Another example is in chat apps where users can send formatted messages with links and images. By inserting HTML right where the user is typing, they can easily format their messages without having to type out all of the HTML tags by hand.In this post, we'll explore various methods to accomplish that goal.
#Inserting a node
To add an element using the
`insertNode()`
function, you can use the following code snippet as an example:js
// Get the current selection
const selection = window.getSelection();
// Get the range of the current selection
const range = selection.getRangeAt(0);
// Create a new element to insert
const newElement = document.createElement('span');
newElement.innerHTML = 'Hello, world!';
// Insert the new element at the current position
range.insertNode(newElement);
In this example, we grab the current selection and range of the selection. Then, we create a new
`span`
element, set its inner HTML to `Hello, world!`
, and insert it at the current position using the `insertNode`
method of the range object.Just keep in mind that this example assumes the contentEditable element is already in focus. Check out the live demo that showcases this approach in action! Don't forget to focus on the content area before clicking the button.
#Using execCommand function
You can also insert HTML at the current position of a contentEditable element by using
`execCommand`
with the `insertHTML`
command. Check out this example:js
// Get the current selection
const selection = window.getSelection();
// Get the HTML to insert
const htmlToInsert = '<div>Hello, world!</div>';
// Insert the HTML at the current position
document.execCommand('insertHTML', false, htmlToInsert);
In this example, we first get the current selection and save it. Then, we define the HTML we want to insert as a string variable. Finally, we use the
`execCommand`
method with the `insertHTML`
command to insert the HTML at the current position.It's important to note that while this method is simpler than creating a new element and inserting it with
`range.insertNode()`
, it may not work in all browsers.The
`execCommand`
method is also useful for formatting selected text. For example, you can make the selected text bold by using the `'bold'`
command.js
// Get the current selection
const selection = window.getSelection();
// Use execCommand to make selected text bold
document.execCommand('bold', false, null);
To create a link around selected text, you can use the
`'createLink'`
command and pass in a URL argument. For example:js
// Get the current selection
const selection = window.getSelection();
// Use execCommand to create a link around selected text
document.execCommand('createLink', false, 'https://phuoc.ng');
This will create a link around any selected text with the provided URL.
#See also
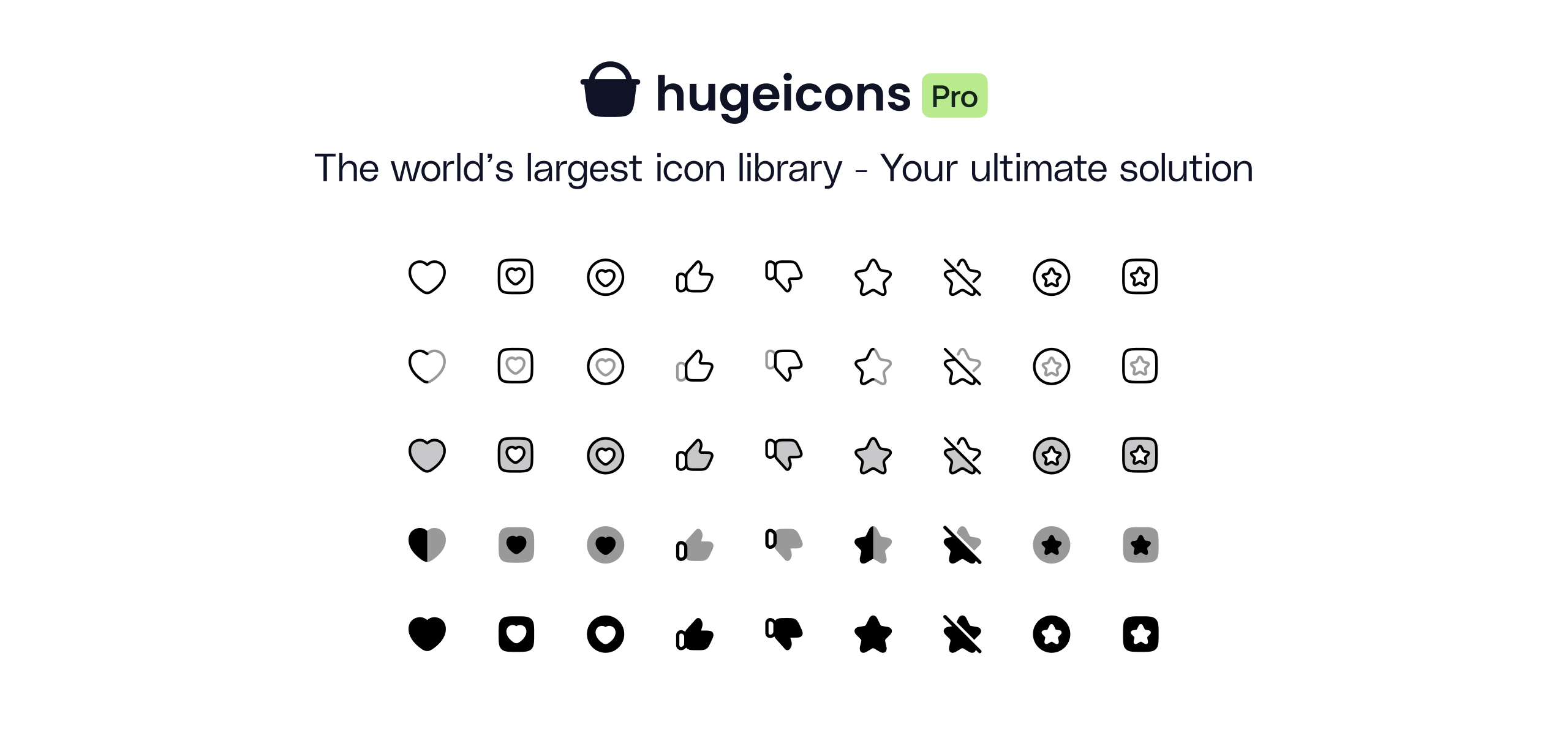
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn