Avoid shifting layout when opening a modal
Written byPhuoc Nguyen
Created
13 Sep, 2023
Category
Tip
When a modal pops up, it's standard practice to disable scrolling on the main page. This keeps users from accidentally clicking on content outside of the modal, which could disrupt their experience.
But here's the thing: hiding the scrollbar can cause layout shift problems. When the scrollbar disappears, it changes the size of the container, which can cause other elements to shift around. This can be especially frustrating if users are already interacting with the page.
To avoid this problem, we need to account for any changes in the container caused by hiding or showing the scrollbar. To do that, we can calculate the width of the scrollbar and make room for it accordingly.
To calculate the width of a scrollbar and adjust for it in the main page, we first need to get the original width of the body element before hiding the scrollbar. We can do this by using
`document.body.offsetWidth`
.Next, we hide the scrollbar by setting
`document.body.style.overflow`
to `hidden`
. Then, we get the new width of the body element, which is affected by hiding the scrollbar. By subtracting this new width from the original width, we can determine the actual width of the scrollbar.js
const currentWidth = document.body.offsetWidth;
document.body.style.overflow = "hidden";
const scrollBarWidth = body.offsetWidth - currentWidth;
To make space for the scrollbar, we need to add its width as a right margin to the body element. We can do this by setting
`document.body.style.marginRight`
to `${scrollBarWidth}px`
. This ensures a smooth user experience by preventing any sudden layout shifts when hiding or showing the scrollbar.js
document.body.style.marginRight = `${scrollBarWidth}px`;
Here's a code snippet you can use to fix this issue when opening a modal:
js
const openModal = () => {
const currentWidth = document.body.offsetWidth;
document.body.style.overflow = "hidden";
const scrollBarWidth = body.offsetWidth - currentWidth;
document.body.style.marginRight = `${scrollBarWidth}px`;
};
Similarly, we reset the
`overflow`
and `margin-right`
properties when we close a modal.js
const closeModal = () => {
document.body.style.overflow = "auto";
document.body.style.marginRight = "";
};
#See also
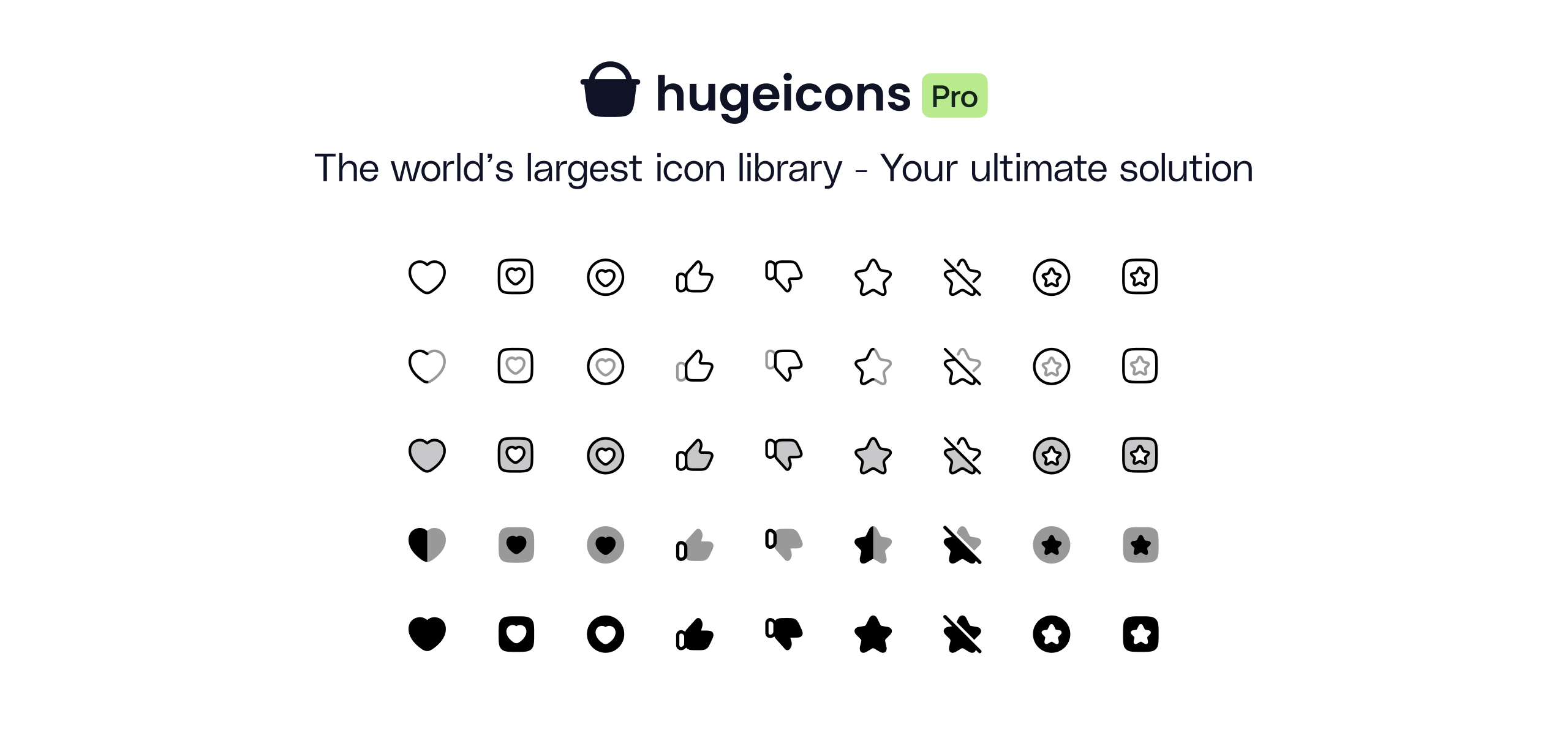
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn