function vs property in interface
Written byPhuoc Nguyen
Created
18 Aug, 2020
Category
TypeScript
There are two ways to define a method in an interface.
- Declare as a property whose type is function
js
interface Logger {
log: (message: string) => void;
}
- Declare as a normal function
js
interface Logger {
log(message: string): void;
}
#Differences
-
If the method is declared as a interface function, then it's possible for you to add more overload versions.jsinterface Logger {log(message: string): void;}// In other placesinterface Logger {log(message: string, level: string): void;}Declaring method as a property, on the other hand, prevents you from duplicating the property declarations which have different types:jsinterface Logger {log: (message: string) => void;}// Does not workinterface Logger {log: (message: string, level: string) => void;}
-
The
`readonly`
modifier only has effect with the property declaration.jsinterface Person {firstName: string;lastName: string;readonly fullName: () => string;// Doesn't work// readonly fullName(): string;} -
TypeScript generates different output for a class that implements the interface methods.Assume that we have a class
`ConsoleLogger`
that simply logs the message in the Console window.For the first approach:jsinterface Logger {log: (message: string) => void;}class ConsoleLogger implements Logger {log = (message: string) => {console.log(message);};}// Generated JavaScript code://// class ConsoleLogger {// constructor() {// this.log = (message) => {// console.log(message);// };// }// }For the second approach:jsinterface Logger {log(message: string): void;}class ConsoleLogger implements Logger {log(message: string) {console.log(message);}}// Generated JavaScript code://// class ConsoleLogger {// log(message) {// console.log(message);// }// }Looking at the generated JavaScript codes, you'll see the different outputs.The first approach produces a property`log`
in the constructor. It means that`log`
will be created every time you create a new instance of class.While the second approach produces the`log`
method, and it exists in all instances of class. The`log`
method also is a member of class prototype, so we can extend the class to override the method if needed:jsclass ConsoleLogger implements Logger {log(message: string) {console.log(message);}}class ConsoleLoggerWithColor extends ConsoleLogger {// Override the `log` methodlog(message: string) {// Display the message in white color and blue background areaconsole.log('%c%s', 'color: white; background: blue', message);}}See the differences between declaring methods in class constructor and prototype for more details.
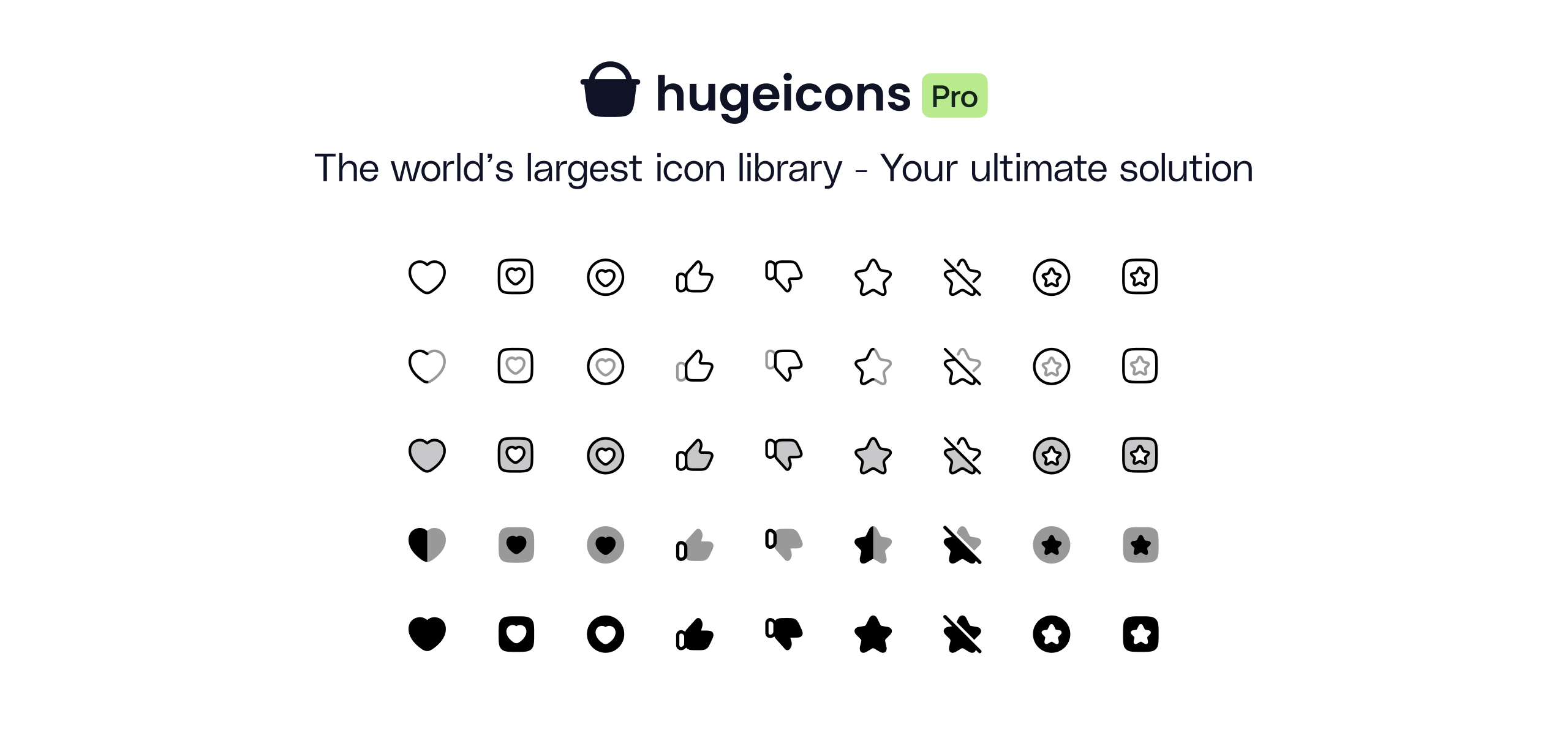
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn