Use a custom drag handle
Written byPhuoc Nguyen
Created
24 Nov, 2023
When designing a web application that requires drag and drop functionality, using a custom drag handle can provide several benefits. For example, in a project management tool where users need to move tasks around, using a custom drag handle can make it easier for users to differentiate between dragging a task and clicking on its content. Developers can also customize the appearance of the handle to match the overall design of the application, providing a more cohesive user experience. Using unique custom drag handles for each draggable element on the page can prevent confusion and improve usability.
Many popular websites and applications, such as Trello, Figma, and Shopify, make use of custom drag handles to improve the user experience. For instance, Trello uses a small horizontal bar on task cards to distinguish between selecting and dragging a card. Figma uses small circles with directional arrows inside them to give users precise control over where they place design elements on their canvas. Shopify's drag and drop website builder, "Sections" employs intuitive drag handles with four directional arrows arranged in a cross shape to help users rearrange page sections with ease.
By incorporating well-designed custom drag handles into web applications, companies can improve both functionality and aesthetics. In this post, we'll learn how to use a custom drag handle to make an element draggable with React.
#Making an element draggable
Let's review how to make an element draggable. To achieve this functionality, we created a reusable hook called
`useDraggable`
.Using the hook is simple. Just call the function and it will return an array of three items. This makes it easy to add draggable functionality to any element you want.
ts
const [draggableRef, dx, dy] = useDraggable();
To move an element around, we need to know three things: which element we want to move, how far it has been dragged horizontally (
`dx`
), and how far it has been dragged vertically (`dy`
) from its starting position. We can select the target element using the `ref`
attribute, and position it within its container using the `translate`
function and the `transform`
property.Here's a quick sample code to refresh your memory:
tsx
<div className="container">
<div
className="draggable"
ref={draggableRef}
style={{
transform: `translate(${dx}px, ${dy}px)`,
}}
/>
</div>
Take a look at the demo below where you can easily move the entire element around your screen just by dragging it.
#Making dragging easier with a custom handle
Sometimes, it's hard for users to tell the difference between clicking on an element and dragging it. This is especially true if the element is small. But don't worry, we have a solution: a custom drag handle.
By adding a child element inside our draggable component, users can click and drag anywhere within the handle element without accidentally triggering other events or clicking outside of the target area.
To implement this, we just need to attach the
`ref`
returned by the `useDraggable`
hook to the handle element instead of the whole element. And we can still use the `dx`
and `dy`
properties to position the whole element.This simple trick will make dragging elements a breeze! Check out this sample code to see it in action:
tsx
const [draggableRef, dx, dy] = useDraggable();
return (
<div className="container">
<div
className="draggable"
style={{
transform: `translate(${dx}px, ${dy}px)`
}}
>
<div
className="draggable__handle"
ref={draggableRef}
>
...
</div>
...
</div>
</div>
);
With these modifications, our draggable component has become more intuitive and user-friendly for everyone.
Take a look at the demo below. You can now easily move the entire element around the screen by simply clicking and dragging the header.
#Conclusion
Incorporating a custom drag handle into a web application can greatly improve the user experience. It helps users differentiate between clicking on an element and dragging it around. By using a reusable hook like
`useDraggable`
, we were able to easily implement this feature in React.Custom drag handles not only improve functionality but also enhance the aesthetics of the web application. They can be customized to match the overall design, creating a cohesive look and feel throughout the application.
In conclusion, using a custom drag handle with React is simple and provides significant usability and design benefits. With just a few tweaks to our draggable component, we created an intuitive and user-friendly interface that makes it easy for users to interact with elements on the page.
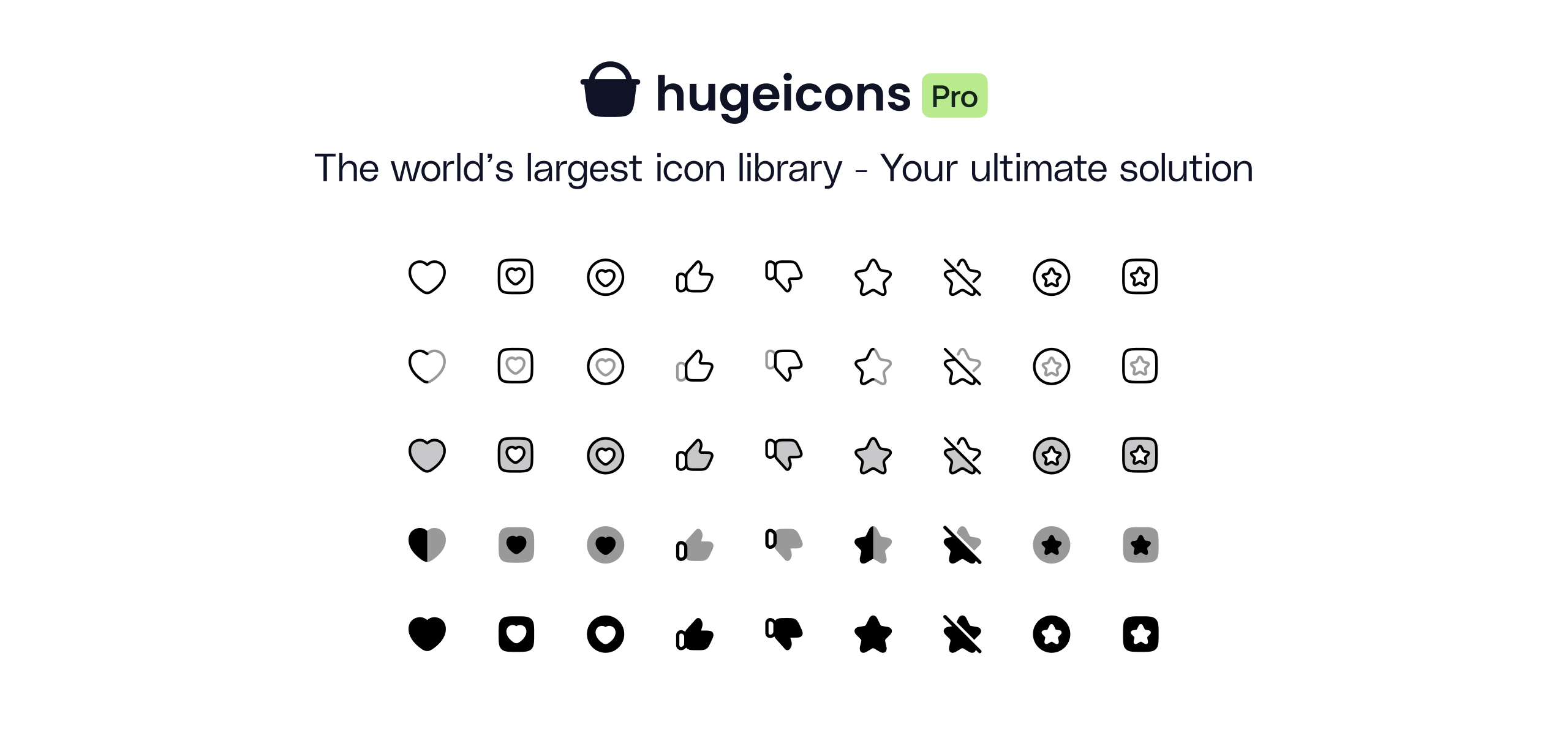
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn