Snap a draggable element to a grid
Written byPhuoc Nguyen
Created
19 Nov, 2023
When we say "snap to grid", we're talking about making a draggable element align to specific points on a grid as it's being moved. This is especially helpful in interfaces where exact positioning matters, like design tools or games. By using a grid and snap-to functionality, you can make sure that elements are always in the right place without needing to adjust them manually.
There are many real-world examples where snapping to a grid is useful. One of these is in creating dashboards or interfaces for data visualization. By snapping elements to a grid, designers can make sure that data points are precisely aligned with each other, which makes it easier for users to compare them.
Snap-to functionality is also helpful in web applications that allow users to create their own content, like designing t-shirts or mugs. By using a snap-to grid, these websites can make sure that the user's design is properly aligned and centered.
Online maps are another example where snapping to a grid is useful. By using a grid-based system, developers can make sure that map markers are placed accurately and don't overlap with each other. This makes it easier for users to navigate the map and find what they're looking for.
Snap-to grids are also used in creating responsive layouts. By snapping elements to specific points on the grid, designers can make sure that their website looks great on different screen sizes without needing to adjust the layout for each size.
Overall, there are countless situations where snapping draggable elements to a grid can be useful in web development. In this post, we'll learn how to create snap-to grid functionality with React.
#Adding precision with a "snap to grid" feature
Adding a "snap to grid" feature to our draggable component can greatly enhance the precision of element positioning for users. This feature aligns the dragged element with a pre-defined grid of cells, allowing users to drop elements into specific locations more easily.
To implement this feature, we must first define a grid size and then snap our element's position to its nearest cell on the grid. We can achieve this by calculating the remainder of our element's position divided by the grid size and adjusting it accordingly.
Previously, we created a reusable hook to make an element draggable. This hook managed an internal state consisting of two properties,
`dx`
and `dy`
, which indicated how far the element had been moved horizontally and vertically.Here's a code snippet we've used to handle the
`mousemove`
event:tsx
const handleMouseMove = (e: React.MouseEvent) => {
// How far the mouse has been moved
const dx = e.clientX - startPos.x;
const dy = e.clientY - startPos.y;
setOffset({ dx, dy });
};
If we want the element to snap to the grid, we can modify our
`handleMouseMove`
function to include snapping.tsx
const handleMouseMove = (e: React.MouseEvent) => {
const dx = e.clientX - startPos.x;
const dy = e.clientY - startPos.y;
// Snap to grid
const gridSize = 50; // Define your own value here
const snappedX = Math.round(dx / gridSize) * gridSize;
const snappedY = Math.round(dy / gridSize) * gridSize;
setOffset({ dx: snappedX, dy: snappedY });
};
In this example, we set our
`gridSize`
to `50`
, but you can use any value that suits your needs. You can even make it a configurable prop of the hook, as shown in the final demo code.Next, we calculate
`snappedX`
and `snappedY`
by rounding our current position divided by `gridSize`
, and then multiplying it back by `gridSize`
. This gives us a new position that aligns perfectly with our grid.By adding this feature, we've made our draggable component even more versatile and user-friendly. Users can now position elements more precisely with less effort, making it easier for them to create the layout they want.
#Adding grid lines to improve user experience
Let's make our design even better by adding some grid lines. This will improve the user experience and make everything look more organized.
To achieve this, we'll use two linear gradients: one for horizontal lines and another for vertical lines.
For the horizontal lines, we'll use
`to right`
to indicate that it should be a gradient that goes from left to right. We'll set the color of the line to `rgb(203 213 225)`
and make it 1 pixel wide. Then, we'll add a transparent space of 1 pixel as well.For the vertical lines, we'll use
`to bottom`
to indicate that it should be a gradient that goes from top to bottom. Again, we'll set the color of the line to `rgb(203 213 225)`
and make it 1 pixel wide with a transparent space of 1 pixel.css
.grid {
background-image:
linear-gradient(to right, rgb(203 213 225) 1px, transparent 1px),
linear-gradient(to bottom, rgb(203 213 225) 1px, transparent 1px);
}
Next, we'll set the size of each background image by using
`background-size: 2.5rem 2.5rem`
. This is important because it tells CSS how big each square on our grid should be.css
.grid {
background-size: 2.5rem 2.5rem;
}
By combining these properties, we can create a grid system that is both simple and effective. This makes it easy for users to snap draggable elements into place.
Take a look at the demo below and try dragging the small rectangle. It will snap right into the grid.
#Conclusion
Creating a snap-to-grid functionality with React is easier than you might think. With just a few lines of code, we can create a custom hook that allows draggable components to snap to a grid. This means users can position elements with greater accuracy, leading to a more polished look and feel.
By using this technique in web development, designers and developers can enhance the user experience by creating visually appealing and user-friendly interfaces. Whether you're building an e-commerce website or a data visualization tool, snapping draggable elements to a grid can help you achieve the perfect layout.
React provides a powerful set of tools for building dynamic and interactive user interfaces. By combining these tools with techniques like snap-to-grid functionality, we can create interfaces that are intuitive and engaging, resulting in a more successful product.
#See also
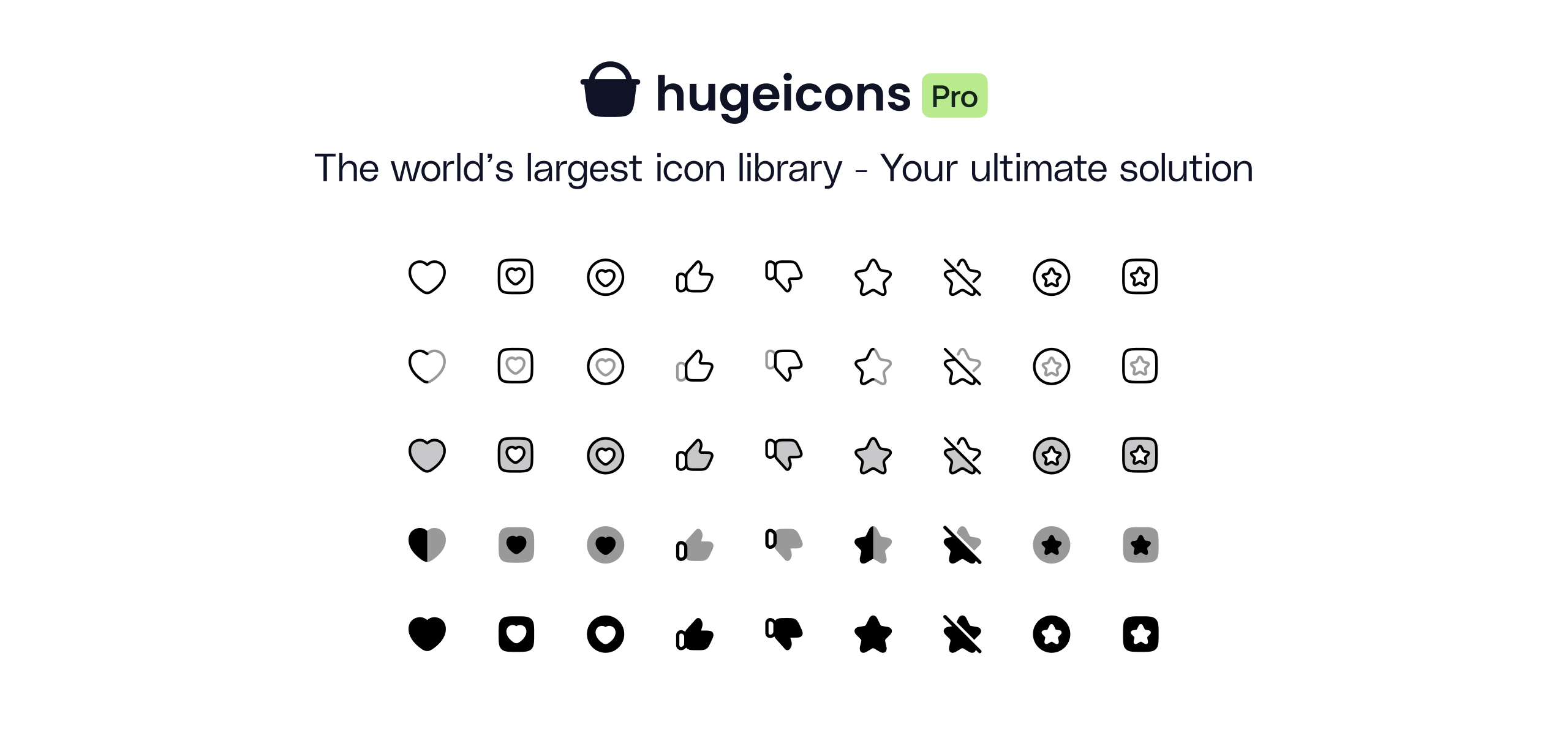
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn