Preview images before uploading them
Written byPhuoc Nguyen
Created
29 Nov, 2023
Uploading multiple images can be a hassle, especially when you have to select each image one by one. But implementing drag and drop functionality can make the process smoother. By allowing users to drag and drop images onto the upload area, you eliminate the need for them to navigate through their file system and select each image individually. This saves time and reduces the potential for mistakes.
Previewing images before uploading is crucial in many scenarios. For example, e-commerce websites often allow customers to upload images of the products they want to sell. It's important to preview the images to ensure that they meet the website's requirements in terms of size and quality.
Social media platforms like Instagram and Facebook also require specific image dimensions, file sizes, and formats. Previewing images before uploading helps users ensure that their pictures look good and meet the platform's requirements.
Many job application forms require candidates to upload their resumes and headshots. In this case, it's important for candidates to preview their headshots before uploading them so that they can ensure that they look professional.
In this post, we'll learn how to use React to provide a preview of images that users drag and drop. This will not only improve the user experience but also reduce errors.
#Handling the drop event
To show a preview of images, we need to handle two drag and drop events. For this, we define two event handlers:
`handleDrop`
and `handleDragOver`
. The `handleDrop`
function is responsible for handling the drop event, while the `handleDragOver`
function handles the dragover event.tsx
<div
onDrop={handleDrop}
onDragOver={handleDragOver}
>
Drag and drop images here
</div>
When a user drags files over the upload area, the
`handleDragOver`
function is called. This function not only handles the drag event, but also prevents the default behavior of opening the file in the browser.ts
const handleDragOver = (e: DragEvent): void => {
e.preventDefault();
};
On the other hand, when a user drops one or more files onto the upload area, the
`handleDrop`
function is called. It stops the browser from opening the dropped file by default.ts
const handleDrop = (e: DragEvent): void => {
e.preventDefault();
// Do something with `e.dataTransfer.files` ...
};
#Previewing dropped images
When files are dropped onto the upload area, we can retrieve information about them through the
`e.dataTransfer.files`
property. This property returns a `FileList`
object that contains details about each dropped file, including the file name, size, type, and last modified date.To preview the images, we'll use an internal state that keeps track of the URL of each image. Initially, this state will be set to an empty array.
ts
const [images, setImages] = React.useState([]);
To process the files transferred, we will loop through the
`FileList`
object provided by the data transfer. For each file, we will check if it is an image by verifying that the `type`
property begins with `image/`
. If it is indeed an image, we will use the `createObjectURL()`
method to read the contents of the file as a data URL.To give you a better idea of how we handle the
`drop`
event, here's a code snippet:ts
const handleDrop = (e: DragEvent): void => {
const files = e.dataTransfer.files;
const imageFiles = files.filter(file => file.type.startsWith('image/'));
if (imageFiles.length) {
const imageURLs = imageFiles.map(imageFile => URL.createObjectURL(imageFile));
setImages(imageURLs);
}
};
In the code snippet below, we check whether users have selected any images. If they have, we loop through them and display each image using the
`img`
tag.tsx
{
images.length === 0
? "Drag and drop images here"
: (
<div>
{
images.map((image) => (
<div key={image}>
<img src={image} />
</div>
))
}
</div>
)
}
We've made an exciting improvement to our system that allows users to preview their images before uploading them. This upgrade not only enhances the user experience but also minimizes the chances of errors in selecting the wrong images for upload.
By providing users with a visual representation of their uploaded images before they are submitted, it enhances their experience and gives them more control over their uploads.
Check out the demo below to see how it works!
#Conclusion
In conclusion, adding drag and drop functionality with image preview can greatly improve the user experience when uploading images. This feature is especially beneficial for e-commerce websites, social media platforms, and job application forms.
Users can now see a visual representation of their uploaded images before submitting them, which enhances their experience and gives them more control over their uploads. Additionally, this feature reduces the chances of errors in selecting the wrong images for upload, which can prevent frustration and save time for both users and developers.
We hope that this post has provided valuable insights into implementing image preview on drag and drop using React. By following the steps outlined here, you can easily add this feature to your own applications and enhance your users' experience.
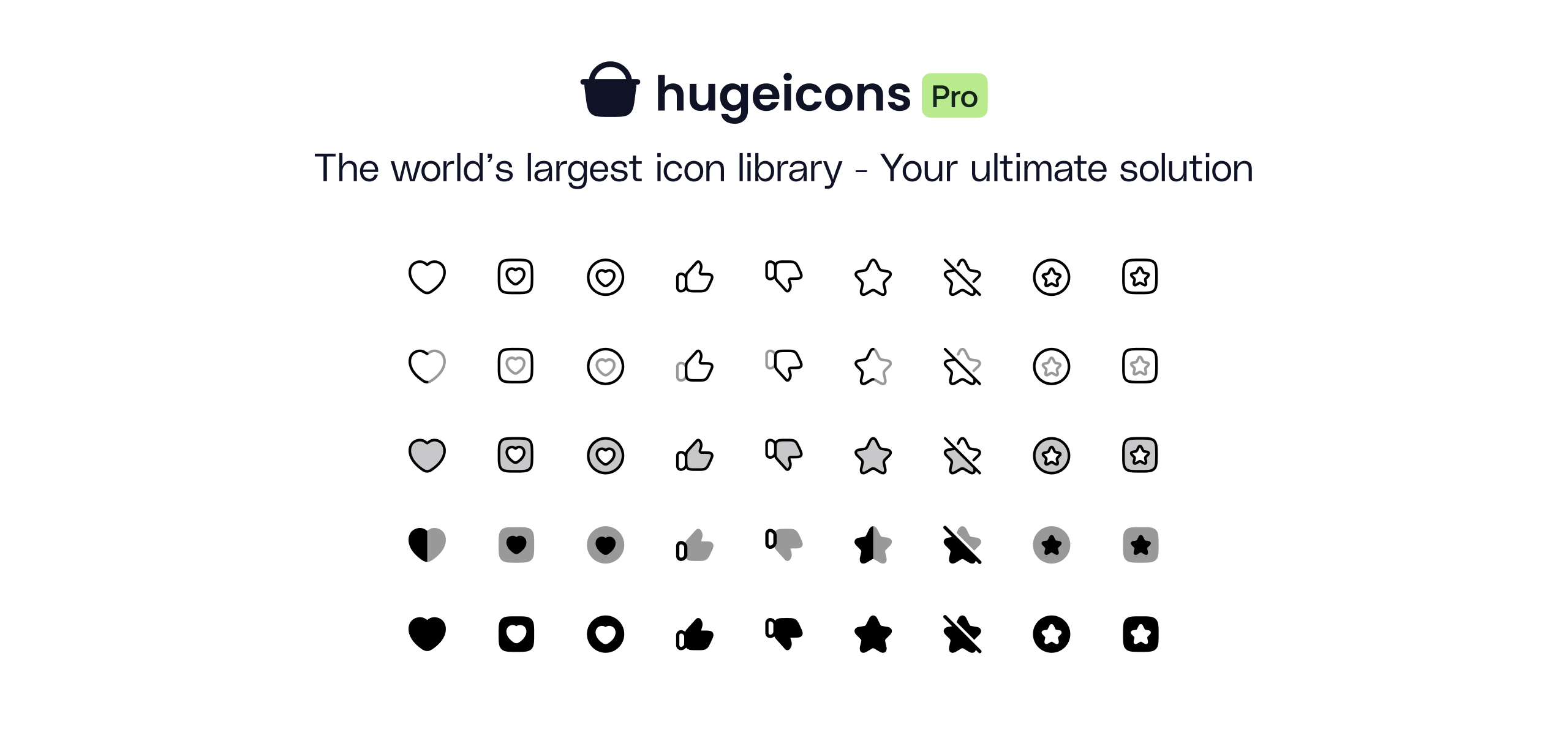
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn