Create an RGB to HEX color converter
Written byPhuoc Nguyen
Created
08 Nov, 2023
Tags
React range slider, RGB to HEX
An RGB to HEX color converter can be a handy tool in many real-life situations. For instance, web developers often use it when designing websites, as it helps them convert RGB colors to HEX codes and use them in their CSS code. This ensures that the website shows the exact colors they want.
Graphic designers also find this tool helpful when working with digital images, as they can quickly convert RGB values to HEX codes for use in their designs. Additionally, artists and photographers may find it useful when editing images or creating digital artwork.
Moreover, individuals looking to personalize the colors of their devices or accessories can use this tool. By converting RGB values to HEX codes, they can input these codes into various settings menus on their devices and achieve a more tailored look and feel.
Overall, an RGB to HEX color converter has many practical applications, making it a valuable tool for anyone working with digital colors.
In our previous post, we learned how to create a range slider with React. In this post, we'll take it a step further and use that range slider to build our own RGB to HEX color converter.
#Displaying the current value
As you may know, an RGB color has three properties: red, green, and blue. Each of these properties is represented by a number between 0 and 255.
To create our converter, we can use three range sliders, allowing users to adjust the value of each property. To make it easier for users to see the current value of each property, we'll add a new element to the knob element.
Here's how we can do it:
tsx
<div className="slider__knob">
<div className="slider__value">...</div>
</div>
To move the slider value below the knob, we use absolute positioning on the value element and set its
`top`
property to `100%`
. We also center it horizontally using the `left: 50%`
and `transform: translateX(-50%)`
properties. This ensures that the value is always centered below the knob, no matter how wide it is.css
.slider__value {
position: absolute;
top: 100%;
left: 50%;
transform: translateX(-50%);
}
To track the current value of our slider, we use an internal state called
`value`
. Additionally, we've defined two constants, `MIN`
and `MAX`
, which represent the minimum and maximum values of the slider. In our case, we've set these values to 0 and 255, which are the possible values for a color property.In your own project, you might want to make
`MIN`
and `MAX`
configurable props of the component, so you can easily adjust the range of the slider to fit your specific needs.ts
const MIN = 0;
const MAX = 255;
const [value, setValue] = React.useState(0);
To determine the current RGB color value in our color converter component, we need to calculate the
`value`
state. We do this by using the minimum and maximum values of the range slider, represented by the `MIN`
and `MAX`
variables for each color property.To start, we calculate the percentage value of how far the knob has moved within the container by using
`delta / containerWidth`
. Then, we multiply this percentage value by the range between `MIN`
and `MAX`
to figure out where our current position falls on that scale. Finally, we add this value to `MIN`
to get our current RGB color value.By updating the
`value`
state whenever the `dx`
changes, our RGB color converter component will respond smoothly and accurately to user input.ts
const updatePosition = (dx) => {
const containerWidth = container.getBoundingClientRect().width;
const delta = Math.min(Math.max(0, dx), containerWidth);
const percent = delta / containerWidth;
const value = Math.ceil(MIN + (MAX - MIN) * percent);
setValue(value);
};
To reveal the selected value, we'll add a new prop. This prop will be a function that gets triggered whenever users adjust the value.
ts
const RangeSlider = ({ onChange }) => {
const updatePosition = (dx) => {
// ...
const value = Math.ceil(MIN + (MAX - MIN) * percent);
setValue(value);
onChange(value);
};
};
Here's how we can use the range slider to adjust values between 0 and 255:
#Setting up the converter
To build the converter, we need two internal states: one to store the current RGB color and another to store the corresponding HEX color.
ts
const [{r, g, b}, setRgb] = React.useState({
r: 0,
g: 0,
b: 0,
});
const [hex, setHex] = React.useState('');
The converter has three range sliders that let you set the value for one of the
`r`
, `g`
, or `b`
properties.When you move a slider, it updates the RGB color in real-time. Here's how you can use the range slider to adjust the value of the
`r`
property:tsx
<RangeSlider onChange={handleChangeR} />
When the user adjusts the value of the red property using the range slider, the
`handleChangeR`
function is triggered. This function takes the new value for `r`
and updates the existing RGB color object using the `setRgb`
function.ts
const handleChangeR = (r) => {
setRgb((rgb) => ({ ...rgb, r }));
};
The
`setRgb`
function is pretty neat. It takes in a callback that gets the previous state of the RGB color object (`rgb`
) and returns a new state with an updated `r`
value. We use the spread operator (`...`
) to copy over all the other properties from the previous state so that we don't lose them.By updating each property of our RGB color as users interact with our range sliders, we can easily convert it to a HEX color later on.
We can use the
`useEffect`
hook to keep an eye on changes in the `r`
, `g`
, and `b`
properties of our RGB color object. Whenever any of these values change, we want to convert the new RGB color to a HEX color and update our internal state accordingly.To do this, we can create a function called
`rgbToHex`
that takes the current values of `r`
, `g`
, and `b`
as arguments and returns their corresponding HEX value.There are a few ways to convert an RGB color to a HEX color. You can find some of these methods in this post.
Once we have our
`rgbToHex`
function, we can call it inside an effect hook that is triggered whenever any of those values change.ts
const rgbToHex = (r, g, b) => {
return `#${((1 << 24) + (r << 16) + (g << 8) + b).toString(16).slice(1)}`;
};
React.useEffect(() => {
const hexColor = rgbToHex(r, g, b);
setHex(hexColor);
}, [r, g, b]);
By using the effect hook to monitor changes in these properties, we can guarantee that our HEX color state is always in sync with the most recent RGB color values. This simplifies the process of showing users the current HEX value and enables us to leverage this converted value in other parts of our application, if necessary.
For instance, we can display the hex color as the value of a read-only textbox.
tsx
<input
defaultValue={hex}
readOnly
type="text"
onFocus={handleFocusHex}
/>
To enhance the user experience, we want to make it easier for users to copy and paste HEX color values. So, when a user clicks on the HEX color input field, we can select all of its contents automatically. We achieve this by using the
`onFocus`
event handler and passing in a function called `handleFocusHex`
.ts
const handleFocusHex = (e) => {
e.target.select();
};
This function takes an
`event`
object (`e`
) and uses the `select()`
method to automatically select all the text inside an input field. By doing this, users can easily copy or edit the HEX color value without having to select it themselves. This creates a smoother user experience overall.Take a look at the demo below. Try dragging the knob in each slider to adjust the value of the
`r`
, `g`
, or `b`
property. The HEX value is updated instantly in the text field.There is room for improvement. Specifically, the converter should allow users to enter the hex color directly in the text field. This would automatically update the values of the R, G, and B properties. We'll let you handle these improvements.
#Conclusion
To summarize, creating an RGB to HEX converter with React is an excellent way to learn how to use range sliders and internal states. By following the steps outlined in this post, you can develop your own converter that enables users to adjust RGB color values and view their corresponding HEX values in real-time.
This tool can be valuable for a wide range of applications, from web design to digital art. Moreover, by utilizing React to build this converter, you will gain valuable experience with one of today's most popular front-end frameworks.
#See also
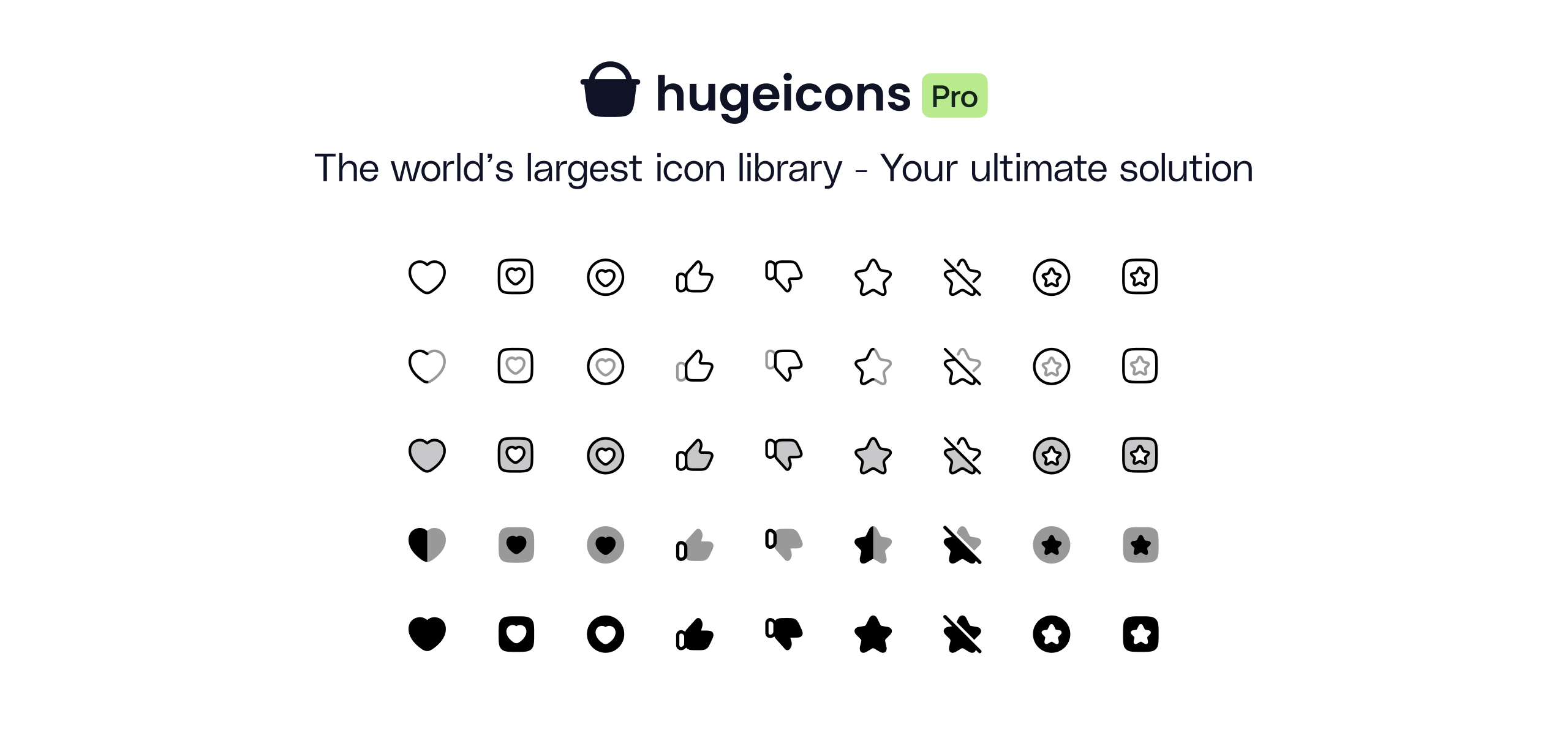
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn