Build a drop zone for file uploads
Written byPhuoc Nguyen
Created
01 Dec, 2023
Tags
Drop zone, FormData
A drop zone is a designated area on a website where users can easily drag and drop files to upload them. It's a simple and intuitive way for users to upload files without navigating through multiple pages or dialogs. Drop zones are becoming increasingly popular as more web applications require file uploads, and they can be easily implemented using HTML5 and JavaScript.
Using a drop zone has several advantages over traditional file upload methods:
- Simplicity: A drop zone provides a straightforward and user-friendly way for users to upload files.
- Convenience: Users can drag and drop files onto the drop zone instead of navigating through multiple pages or dialogs.
- Flexibility: Drop zones can accept any type of file, making it easy for users to upload documents, images, videos, and other types of content.
- Interactivity: Drop zones can provide feedback to users as they drag and drop files, such as highlighting the area where the file will be dropped or displaying a progress bar during the upload process.
Overall, implementing a drop zone on your website can greatly improve the user experience when it comes to uploading files.
Drop zones can be helpful in many real-life situations. For example, popular cloud storage services like Dropbox and Google Drive use drop zones as the main way to upload files to your account.
E-commerce websites often use drop zones for customers to upload product images or their own designs for custom products.
Platforms like Instagram or YouTube, where users share photos or videos, can use drop zones to make uploading content easier.
Web applications that require file uploads, such as project management tools or document editors, can also benefit from using a drop zone as it provides a simple and intuitive way for users to upload files.
In this post, we'll learn how to build a drop zone and submit the dropped files to a server with React. By the end of this post, you'll be able to create a functional and visually appealing drop zone.
#Simplify file uploads with drag and drop
Uploading files to a website can be a hassle. But with drag and drop events, we can provide users with an easy and intuitive way to upload files directly from their web browser.
To get started, we need to create a drop zone on our web page where users can drop their files. This is easy to do by adding an event listener for the
`dragover`
event on the element we want to use as the drop zone.DropZone.tsx
const handleDragOver = (e) => {
e.preventDefault();
// Add styles to indicate that a file can be dropped here
};
// Render
<div onDragOver={handleDragOver}>
...
</div>
To avoid the browser's attempt to open or download dragged files, we prevent the default behavior of the
`dragover`
event.Once we've accomplished that, we move on to handling the
`drop`
event. This is where we retrieve the dropped file(s) and send them to our server.DropZone.tsx
const handleDrop = (e) => {
e.preventDefault();
// Do something with `e.dataTransfer.files`
};
// Render
<div onDrop={handleDrop}>
...
</div>
In this example, we first need to prevent the default behavior of the
`drop`
event. This ensures that the browser won't try to open or download any files.Once users drop files onto the designated zone, we can retrieve information about them using the
`e.dataTransfer.files`
property. This property returns a `FileList`
object that contains details about each dropped file, such as the file name, size, type, and last modified date.In the next section, we'll dive into how to use
`FormData`
and `fetch`
API to send the dropped files to the server.#An overview of FormData
If you're building a web application that needs to submit form data to a server, you'll want to know about
`FormData`
. This built-in JavaScript object is a handy tool for constructing key-value pairs that represent form fields and their values. You can use it to easily send data to a server via AJAX requests or the `fetch`
API.To get started with
`FormData`
, simply create an instance of it by calling its constructor method.js
const formData = new FormData();
Next, you can add form fields to it using either the
`append()`
or `set()`
method. The `append()`
method adds a new value to a field if one already exists, otherwise it creates a new field. On the other hand, the `set()`
method replaces any existing value for that field.js
formData.append('username', 'johndoe');
formData.append('email', 'johndoe@example.com');
formData.set('password', '...');
After you've added all the necessary fields, you can send them over HTTP using the
`fetch`
API.js
fetch('/api/user', {
method: 'POST',
body: formData
}).then((response) => {
console.log(response);
});
#Uploading files with fetch API
If you're working with forms that include file uploads, you'll need to handle the binary data associated with the file. Fortunately,
`FormData`
makes this process a breeze.With
`FormData`
, you can easily send files from the client-side to the server using the fetch API. This feature has been available since HTML5 and greatly simplifies the entire process of handling file uploads. By using `FormData`
in conjunction with drag and drop events, users can simply drag and drop files onto an upload area on a web page, which will trigger an event that sends the files to the server for processing.The
`fetch`
API provides a modern way to make HTTP requests from the browser, including the ability to upload files. To upload files using `fetch`
, you can simply pass a `FormData`
object as the `body`
of your request.Here's an example of how to use fetch to upload a file:
js
const formData = new FormData();
for (const file of e.dataTransfer.files) {
formData.append('file', file);
}
fetch('/api/upload', {
method: 'POST',
body: formData
}).then((response) => {
console.log(response);
});
In this example, we start by creating a new
`FormData`
instance. Then, we loop through the files that were dropped using the `dataTransfer.files`
property, and add each of them to the `FormData`
instance. Finally, we create a POST request using `fetch`
and pass in the `FormData`
object as the body.When the request is sent, the server receives the uploaded file in its raw binary form as part of the request body. The server can then process this data and save it to disk or perform other operations on it.
Using
`fetch`
with `FormData`
makes it super easy to handle file uploads in web applications without the need for complex libraries or plugins. With just a few lines of code, we can provide users with an intuitive drag and drop interface for uploading files directly from their browsers.#Dealing with file upload errors
During file uploads, errors can occur for various reasons. For instance, the server may fail to process the uploaded file due to connectivity issues or insufficient storage space. In such cases, it's crucial that our application handles these errors gracefully and communicates them effectively to the user.
To handle file upload errors, we can add a
`catch`
block after sending the request using the `fetch`
API. This catch block will capture any errors thrown during the request and allow us to handle them appropriately. For example, we can display an error message on the UI indicating that something went wrong.Here's an example of how we can handle file upload errors using
`fetch`
:js
fetch('/api/upload', {
method: 'POST',
body: formData
}).then((response) => {
console.log(response);
}).catch((error) => {
console.error(error);
// Display an error message on the UI ...
});
If an error occurs during a request, the
`catch`
block catches it and logs it to the `console`
. We can then display a clear error message on the UI to let users know what happened.By handling errors smoothly during file uploads, we can enhance the user experience of our web application. This ensures that users are aware of any problems that may arise while they interact with our application.
Security considerations for file uploads
When it comes to file uploading using FormData and fetch API, we need to be aware of potential security risks. One of the biggest risks is the possibility of malicious files being uploaded to our servers.
To minimize these risks, there are several security measures we can implement:
Validating file types
We can validate the type of file being uploaded on both the client-side and server-side. By checking the MIME types or file extensions, we can ensure that only valid files are uploaded. If an invalid file type is detected, we can reject it before it reaches our server.
Setting file size limits
We can set limits on the size of files that users are allowed to upload. This helps prevent large files from causing performance issues or overwhelming our servers. It can also help prevent DoS attacks where attackers try to flood our server with large amounts of data.
Filtering on the server-side
We should filter out any potentially harmful content, such as scripts or executable code, in uploaded files on the server-side. We can do this by scanning each uploaded file for known vulnerabilities or exploits before storing them on our servers.
Using HTTPS encryption
Using HTTPS encryption for all communication between clients and servers ensures that data transmitted during file uploads cannot be intercepted or tampered with by third parties.
By implementing these security measures, we can significantly reduce the risks associated with file uploading in our web applications. It's important to note that no single measure alone is enough. Instead, we should use a combination of techniques to provide comprehensive protection against threats.
#Conclusion
In conclusion, using HTML5 drag and drop is an intuitive and user-friendly way for people to upload files directly from their browsers. This feature allows users to easily drag and drop files from their desktop or file explorer directly into the web application, without having to navigate through file dialogs. It's a powerful feature that can greatly improve the user experience of web applications.
We can send files from the client-side to the server using
`FormData`
and `fetch`
, without having to navigate through file dialogs. These APIs provide a simple and effective way to handle user-generated content while keeping the web application fast and responsive.Overall, using HTML5 drag and drop along with
`FormData`
and `fetch`
is a powerful combination that simplifies the process of uploading files. It improves the user experience of our web applications. By implementing this feature and taking the necessary security measures, we can provide a seamless and secure file uploading experience for our users.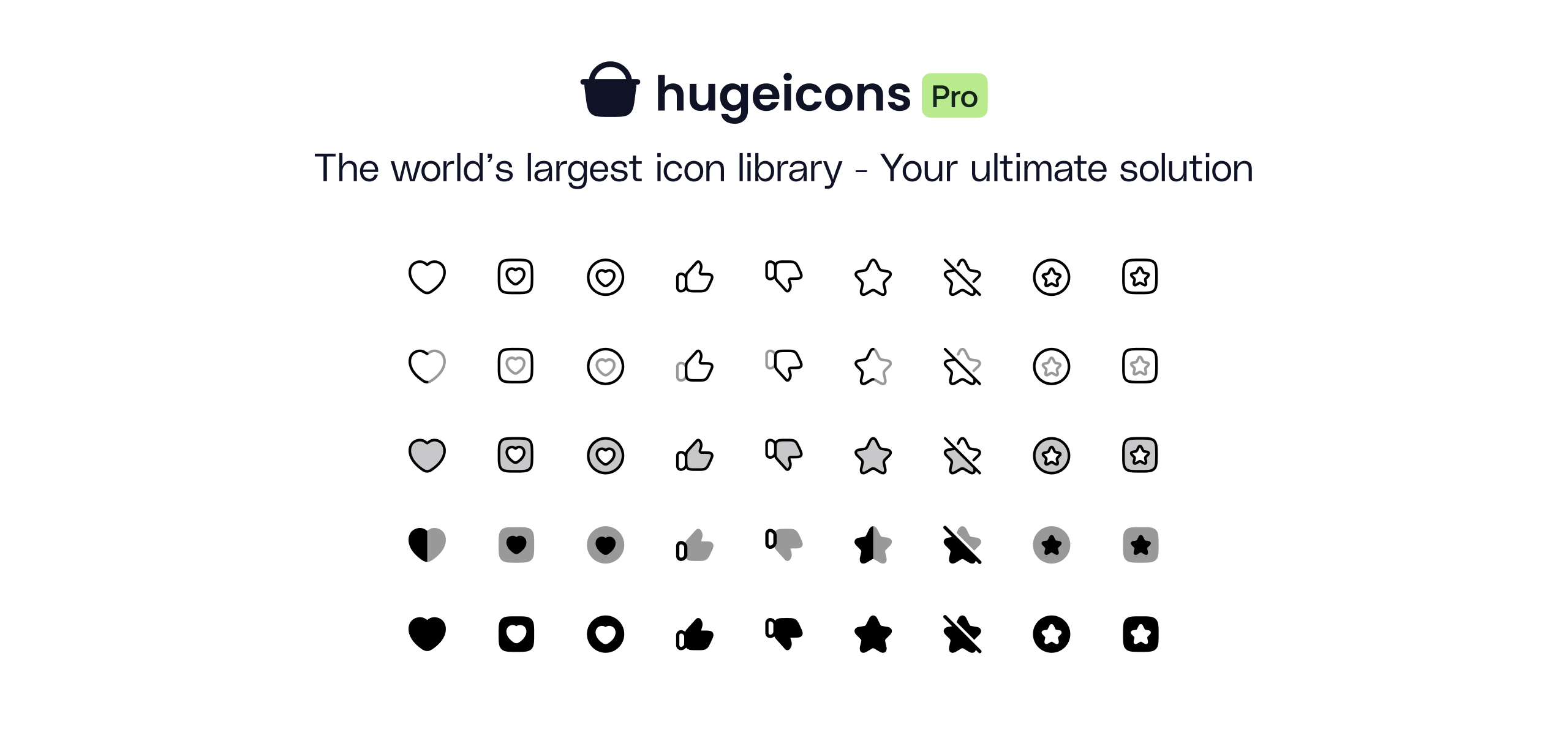
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn