Move the cursor to the end of a contentEditable element
Written byPhuoc Nguyen
Created
18 Sep, 2023
Category
Level 1 — Basic
At times, you may need to move the cursor to the end of a contenteditable element. This can come in handy when you want to focus on an empty element or add new content at the end.
For instance, imagine a chat application where users can reply to a message. It would be great if the cursor automatically moved to the end of the input field, which would already contain the original message, every time they start replying. This way, users don't have to manually move the cursor each time they want to type something new.
Another example is when you're building an editor. In this case, it's crucial that the cursor always stays at the end of the text so that users can continue typing without worrying about moving the cursor around. This can save them a lot of time and effort, and make their overall experience much smoother.
There are also situations where you might want to move the cursor to the end of an element programmatically. For example, if you have an input field with some text, but you want to add more text dynamically using JavaScript, you will need to move the cursor to the end of the input field before adding new text.
To move the cursor to the end of a contenteditable element, we can use a
`Range`
object. We simply set the start and end points of the range to be at the very end of our element, and then add this range to our selection using `window.getSelection().addRange()`
.Here's a breakdown of the steps involved:
js
const moveCursorToEnd = () => {
const range = document.createRange();
const selection = window.getSelection();
range.setStart(contentEle, contentEle.childNodes.length);
range.collapse(true);
selection.removeAllRanges();
selection.addRange(range);
};
To start, we create a new
`Range`
object by calling `document.createRange()`
. Then, we position the start of the range at the very end of our `contentEle`
node by setting its starting node as `contentEle`
and its offset as `contentEle.childNodes.length`
.Next, we collapse the range to ensure that only one point in our contenteditable element is selected. We do this by calling
`range.collapse(true)`
, which sets the endpoint equal to the start point.Finally, we remove any existing ranges from our selection by calling
`sel.removeAllRanges()`
. Then, we add our newly created range to the selection using `sel.addRange(range)`
.#See also
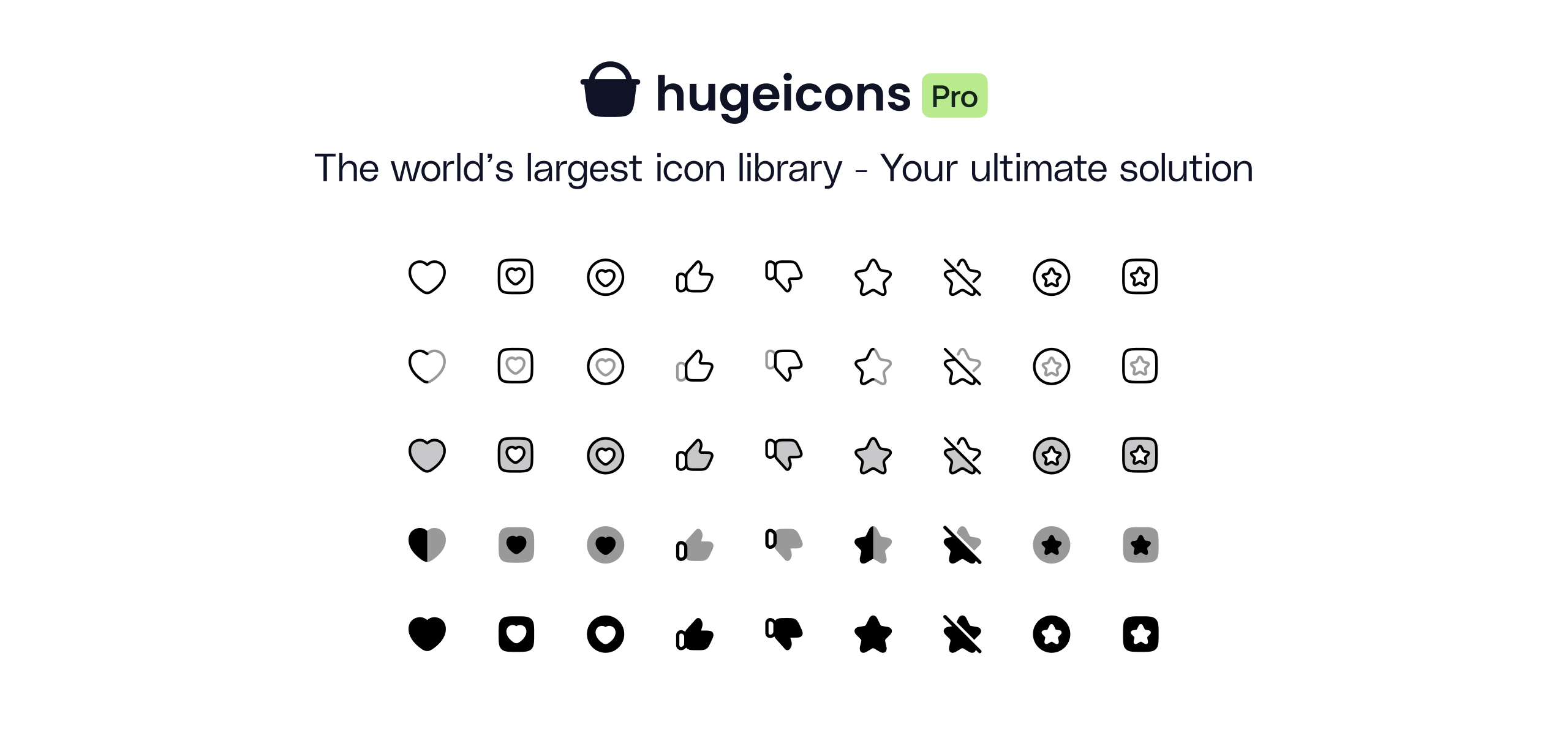
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn