Get size of the selected file
Written byPhuoc Nguyen
Created
10 Mar, 2020
Last updated
07 May, 2021
Category
Level 1 — Basic
In the markup below, we have two elements defined by different
`id`
attributes.
The `id="size"`
element will be used to display the size of selected file from the `id="upload"`
element.html
<input type="file" id="upload" />
<div id="size"></div>
We listen on the
`change`
event of the file input, and get the selected files via `e.target.files`
.
The file size in bytes of the selected file can be retrieved from the `size`
property of the first (and only) file.The size element is shown up or hidden based on the fact that user selects a file or not.
js
// Query the elements
const fileEle = document.getElementById('upload');
const sizeEle = document.getElementById('size');
fileEle.addEventListener('change', function (e) {
const files = e.target.files;
if (files.length === 0) {
// Hide the size element if user doesn't choose any file
sizeEle.innerHTML = '';
sizeEle.style.display = 'none';
} else {
// File size in bytes
sizeEle.innerHTML = `${files[0].size} B`;
// Display it
sizeEle.style.display = 'block';
}
});
#Display a readable size
There is a room for improving the output of file size. Instead of displaying in bytes, we can transform it to a readable format in kB, MB, GB, and TB depending on how big it is.
The following
`formatFileSize`
helper method is created for that purpose:js
// Convert the file size to a readable format
const formatFileSize = function (bytes) {
const sufixes = ['B', 'kB', 'MB', 'GB', 'TB'];
const i = Math.floor(Math.log(bytes) / Math.log(1024));
return `${(bytes / Math.pow(1024, i)).toFixed(2)} ${sufixes[i]}`;
};
// Display the file size
sizeEle.innerHTML = formatFileSize(files[0].size);
#Use case
- Validate the file size before uploading to the server.
#Demo
#See also
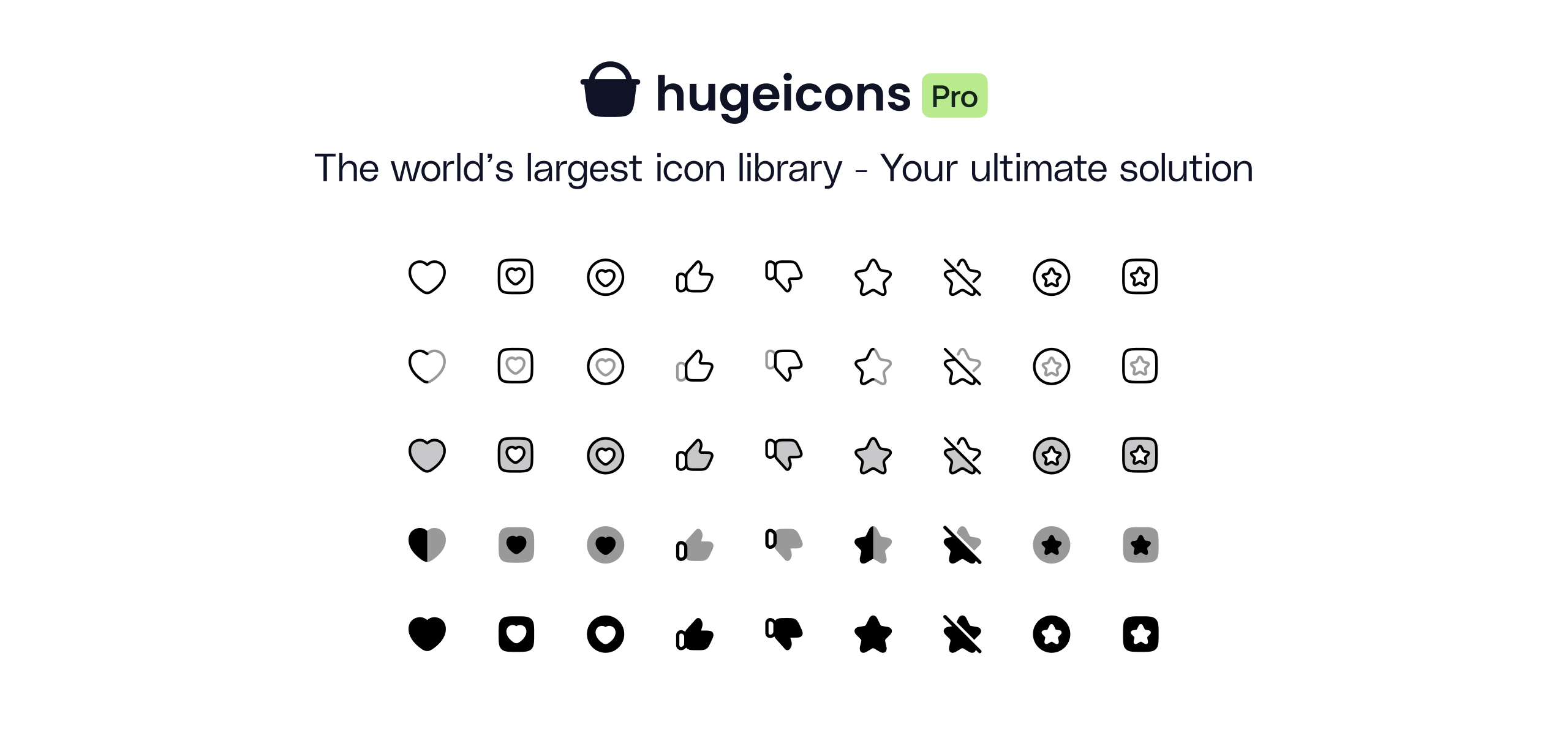
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn