Detect if users open another tab of the current page
Written byPhuoc Nguyen
Created
18 Sep, 2023
Category
Level 3 — Advanced
Have you ever used an online application that only allows you to use it in a single tab? If you try to access the app in another browser tab, you'll be notified and asked to close the newly opened tab.
In this post, I'll show you how to use JavaScript to create the same functionality. You can also apply this approach to build web applications that need real-time communication between different tabs or windows.
Let's get started!
#Using the Page Visibility API
The Page Visibility API is a straightforward tool that can help you determine if a user has opened another tab of the current page. This API is compatible with most modern browsers, such as Chrome, Firefox, Safari, and Edge.
To use the Page Visibility API, take a look at this example:
js
// Listen for visibility change events
document.addEventListener('visibilitychange', function() {
// Check if the page is hidden
if (document.hidden) {
console.log('User opened another tab');
} else {
console.log('User is on this tab');
}
});
In this example, we're listening for the
`visibilitychange`
event. This event is triggered when the user either opens another tab or switches back to the current tab. We then check if the `document.hidden`
property is `true`
or `false`
. If it's `true`
, then we know that the user has opened another tab, and we log a message to the console.#Notifying users of new tabs
To notify other tabs that a user has opened a new tab, we can use the
`BroadcastChannel`
API. This feature allows us to send messages between browsing contexts (tabs) with the same origin. Here's an example of how to use the `BroadcastChannel`
API:js
// Create a new BroadcastChannel with a unique channel name
const channel = new BroadcastChannel('tab-activity');
// Listen for messages on the channel
channel.addEventListener('message', (event) => {
if (event.data === 'open-new-tab') {
console.log('User opened another tab');
}
});
// Send a message to all other tabs when a new tab is opened
document.addEventListener('visibilitychange', () => {
if (document.hidden) {
channel.postMessage('open-new-tab');
}
});
In this example, we create a new
`BroadcastChannel`
with a unique name and listen for messages on that channel. When the user opens another tab, we send a message to all the other tabs using the `BroadcastChannel`
. When a tab receives this message, it logs a message to the console indicating that the user has opened another tab.#Notifying users when closing a tab
To let users know when they're about to close a tab, we can use the
`beforeunload`
event. This event is triggered when the user navigates away from the current page or tries to close the current tab. Here's an example of how to use the `beforeunload`
event:js
const channel = new BroadcastChannel('tab-activity');
window.addEventListener('beforeunload', function(event) {
// Send a message to all other tabs that this tab is closing
channel.postMessage('tab-closing');
});
In this example, we're listening for the
`beforeunload`
event. This event is triggered when the user closes the current tab or navigates away from the current page. Once the event is detected, we create a new `BroadcastChannel`
and send a message to all other tabs, letting them know that this tab is closing.Let's have some fun with the demo below. Follow these steps to see an updated message in the center:
- Duplicate this tab
- Return to this tab when the new one opens
- Close the newly opened tab and watch the magic happen.
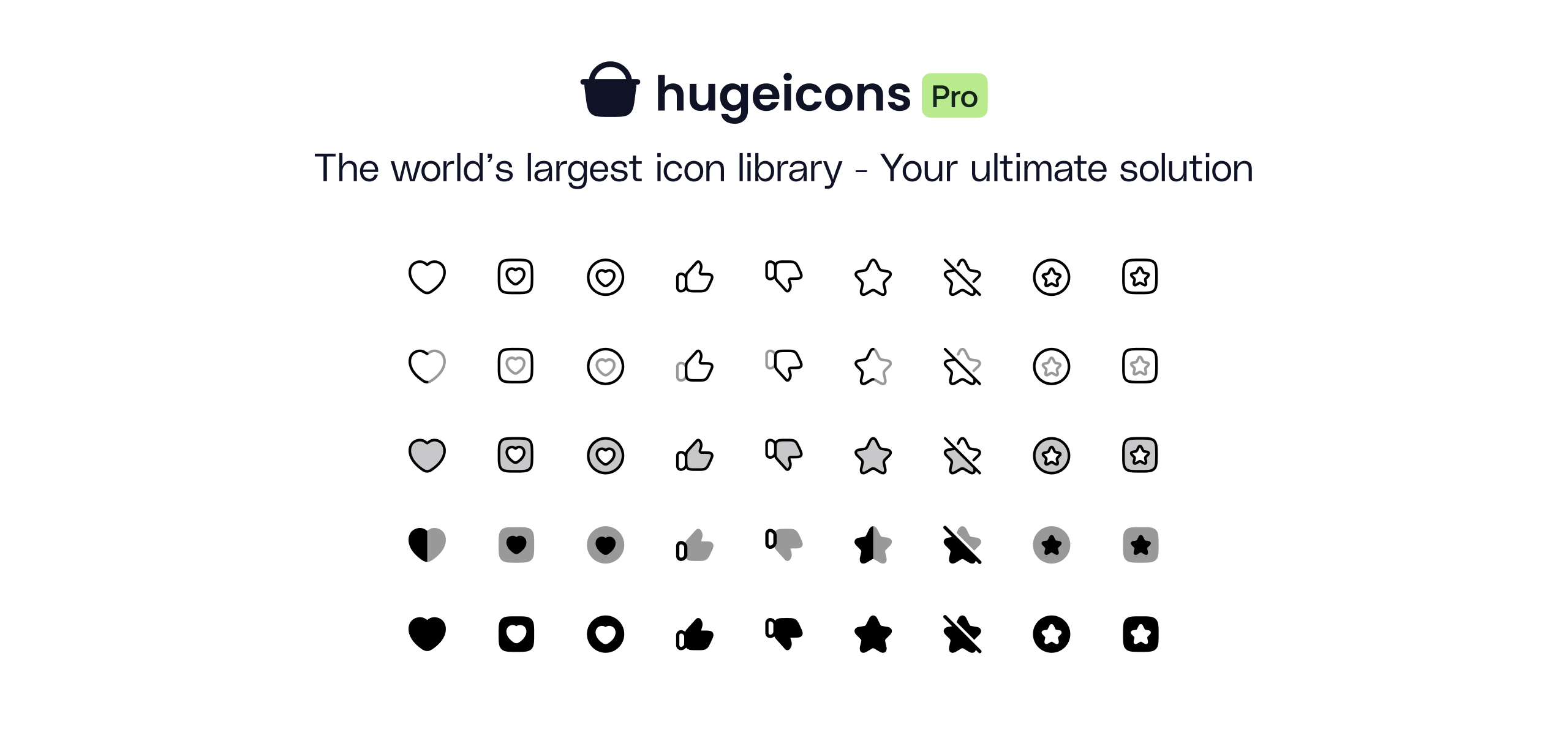
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn