Calculate the reading time of a webpage
Written byPhuoc Nguyen
Created
23 Sep, 2023
Category
Level 1 — Basic
As a web developer, you might want to let users know how long it will take to read the content on your webpage. This is especially helpful if they're in a hurry. In this post, we'll show you how to use JavaScript to calculate the reading time of a webpage.
First, we need to figure out the average reading speed of our users. According to research, most adults read at a speed of about 200-300 words per minute (WPM). However, this can vary depending on factors like age, language, and reading comprehension.
For this post, we'll use an average reading speed of 250 WPM.
Next, we need to count the number of words on the webpage. We can do this using JavaScript by selecting the element that contains the content and using the
`textContent`
property to get the text. Then, we can split the text into an array of words with the `split()`
method and count the number of words with the `length`
property.Here's an example of the code:
js
const content = document.querySelector('#content');
const words = content.textContent.split(' ');
const numWords = words.length;
Now that we have the average reading speed and word count, we can calculate the reading time. We can do this by dividing the word count by the average reading speed and rounding up to the nearest minute.
Here's a code example:
js
// Words per minute
const readingSpeed = 250;
const readingTime = Math.ceil(numWords / readingSpeed);
Finally, we can display the reading time to the user. We can select an element on the page to display the reading time and set its
`textContent`
property to the calculated reading time.Here's an example of the code in action:
js
const readingTimeEle = document.querySelector('#reading-time');
readingTimeEle.textContent = `${readingTime} minute read`;
#Conclusion
In this post, we've learned how to calculate how long it takes to read a webpage using JavaScript. By giving users an estimate of how much time they'll need to read your content, you can improve their experience and help them manage their time more effectively.
#See also
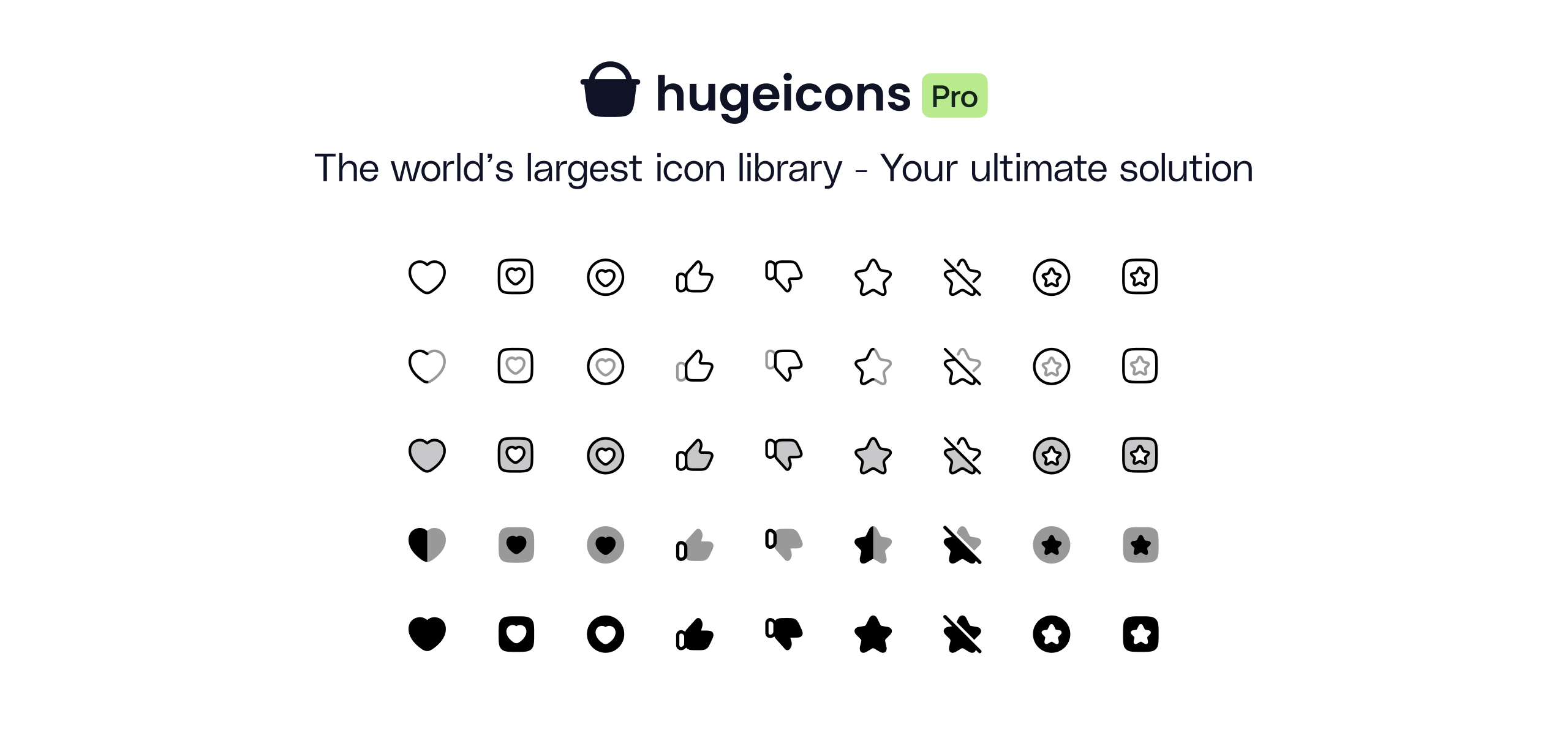
Questions? 🙋
Do you have any questions about front-end development? If so, feel free to create a new issue on GitHub using the button below. I'm happy to help with any topic you'd like to learn more about, even beyond what's covered in this post.
While I have a long list of upcoming topics, I'm always eager to prioritize your questions and ideas for future content. Let's learn and grow together! Sharing knowledge is the best way to elevate ourselves 🥷.
Recent posts ⚡
Newsletter 🔔
If you're into front-end technologies and you want to see more of the content I'm creating, then you might want to consider subscribing to my newsletter.
By subscribing, you'll be the first to know about new articles, products, and exclusive promotions.
Don't worry, I won't spam you. And if you ever change your mind, you can unsubscribe at any time.
Phước Nguyễn